You want something like:
pdb.gimp_drawable_curves_spline(layer,HISTOGRAM_VALUE,8,[0, 0, 0.37477797513321481, 0.12890625, 0.62344582593250431, 0.8828125, 1, 1])
Trick
You can let Gimp determine the values:
Use the GUI and apply the Curves tool on a sample:
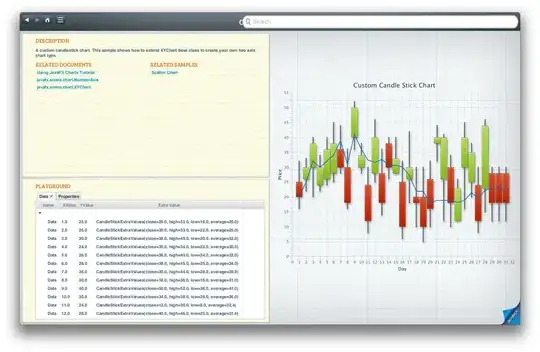
In the image above notice the "Presets" selector at the top. Each time you apply the filter, Gimp saves the curve, and you can retrieve it with the selector. The curve is anonymous by default (only bears a time stamp) but you can also give it a name (click the +
button).
These values are saved in a file in the Gimp profile. In Gimp 2.10 this file is ${Gimp profile}/filters/GimpCurvesConfig.settings
. If you edit it (your editor may need to support long lines), you will see:
# settings
(GimpCurvesConfig "2019-11-08 21:57:15"
(time 1573246635)
(linear no)
(channel value)
(curve
(curve-type smooth)
(points 8 0 0 0.37477797513321481 0.12890625 0.62344582593250431 0.8828125 1 1)
(point-types 4 smooth smooth smooth smooth)
[...snip...]
The line of interest is the one that starts with (points ...
). You just need to transform:
(points 8 0 0 0.37477797513321481 0.12890625 0.62344582593250431 0.8828125 1 1)
into:
... 8,[0, 0, 0.37477797513321481, 0.12890625, 0.62344582593250431, 0.8828125, 1, 1]
Can hardly be easier.
Note:
The term "Bézier curves" is quite incidental here. It is just a way for the software to smooth the curve, but you only set anchor points. The important thing is that you define a function of a channel over itself: newChannelValue=f(oldChannelvalue)
and define f()
not with a mathematical formula but by drawing the graph of the function.