trying to send a message to a group using the Azure Signal R Serverless
You can refer to this github repo that shows with sample code how to implement group broadcasting functionality in Azure functions with Azure SignalR Service.
Add user to a group using the SignalRGroupAction
class
return signalRGroupActions.AddAsync(
new SignalRGroupAction
{
ConnectionId = decodedfConnectionId,
UserId = message.Recipient,
GroupName = message.Groupname,
Action = GroupAction.Add
});
On client side, make request to endpoint to add a user to a group
function addGroup(sender, recipient, connectionId, groupName) {
return axios.post(`${apiBaseUrl}/api/addToGroup`, {
connectionId: connectionId,
recipient: recipient,
groupname: groupName
}, getAxiosConfig()).then(resp => {
if (resp.status == 200) {
confirm("Add Successfully")
}
});
}
Test Result
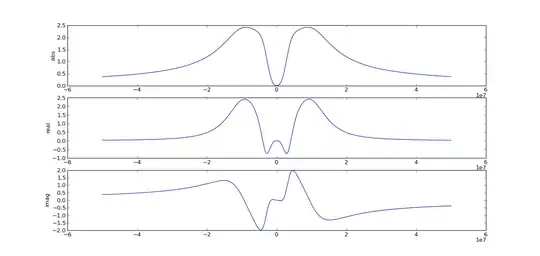
Updated:
Q: "send the message from the JS Client straight from the socket".
A: From here, we can find:
Although the SignalR SDK allows client applications to invoke backend logic in a SignalR hub, this functionality is not yet supported when you use SignalR Service with Azure Functions. Use HTTP requests to invoke Azure Functions.