TL;DR
Using new Date() is faster, both methods are reliable - tested on Chrome, Firefox, Safari.
I wrote test cases on 5 years for the 2 functions.
Then I have run benchmark tests on Chrome, Safari, and Firefox to compare them, here are the results:
https://jsperf.com/string-to-date-regexp-vs-new-date/1
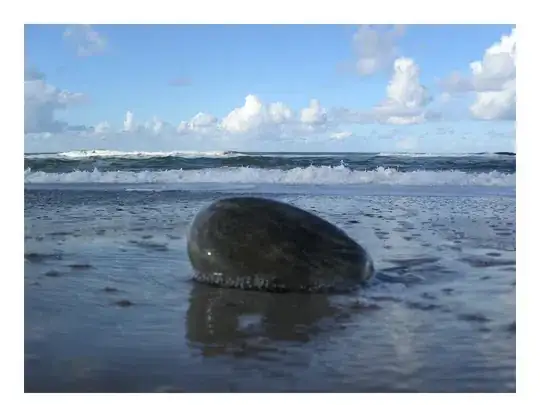
Same results on jsbench.me:
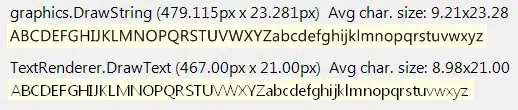
We can see that using regexp is slower for all the cases, (and that Safari is slower than other browsers for this operation).
I also added a check in both cases to validate that the calculated date from stringToDate function is always correct on 5 years (that obviously crosses 10 DLS dates and at least 1 leap year).
If the returned date would be erroneous, the loop would have ended in an error, here are my tests:
- Via regexp
const stringToDate = string => {
const [, y, m, d, h = 0, min = 0] = string.match(/(\d{4})-(\d{2})-(\d{2})(?: (\d{2}):(\d{2}))?/)
return new Date(y, parseInt(m) - 1, d, h, min)
}
let tmpDate = new Date()
for (let i = 0; i <= 365 * 5; i++) {
let y = tmpDate.getFullYear()
let m = tmpDate.getMonth()
let d = tmpDate.getDate()
tmpDate = new Date(y, m, d + 1, 0, 0)
y = tmpDate.getFullYear()
m = tmpDate.getMonth() + 1
d = tmpDate.getDate()
const tmpDateFormatted = `${y}-${m < 10 ? '0' : ''}${m}-${d < 10 ? '0' : ''}${d}`
const calculatedDate = stringToDate(tmpDateFormatted)
// console.log(calculatedDate)
if (calculatedDate.getTime() !== tmpDate.getTime()) {
console.error('Wrong date.', calculatedDate, 'should be', tmpDate)
}
}
- Via new Date()
// Replace '-' with '/' for Safari.
const stringToDate = string => new Date(string.replace(/-/g, '/'))
let tmpDate = new Date()
for (let i = 0; i <= 365 * 5; i++) {
let y = tmpDate.getFullYear()
let m = tmpDate.getMonth()
let d = tmpDate.getDate()
tmpDate = new Date(y, m, d + 1, 0, 0)
y = tmpDate.getFullYear()
m = tmpDate.getMonth() + 1
d = tmpDate.getDate()
const tmpDateFormatted = `${y}-${m < 10 ? '0' : ''}${m}-${d < 10 ? '0' : ''}${d}`
const calculatedDate = stringToDate(tmpDateFormatted)
// console.log(calculatedDate)
if (calculatedDate.getTime() !== tmpDate.getTime()) {
console.error('Wrong date.', calculatedDate, 'should be', tmpDate)
}
}
Hope it helps someone!