You may use
(?i)\b(?<w>\p{L}+)(?:\P{L}+(?<w>(\p{L})(?<=\1\P{L}+\1)\p{L}*))+\b
See the regex demo. The results are in Group "w" capture collection.
Details
\b
- a word boundary
(?<w>\p{L}+)
- Group "w" (word): 1 or more BMP Unicode letters
(?:\P{L}+(?<w>(\p{L})(?<=\1\P{L}+\1)\p{L}*))+
- 1 or more repetitions of
\P{L}+
- 1 or more chars other than BMP Unicode letters
(?<w>(\p{L})(?<=\1\P{L}+\1)\p{L}*)
- Group "w":
(\p{L})
- a letter captured into Group 1
(?<=\1\P{L}+\1)
- immediately to the left of the current position, there must be the same letter as captured in Group 1, 1+ chars other than letters, and the letter in Group 1
\p{L}*
- 0 or more letters
\b
- a word boundary.
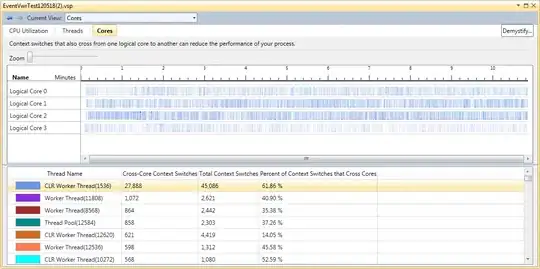
C# code demo:
var text = "One word, duel. Limes said bye.";
var pattern = @"\b(?<w>\p{L}+)(?:\P{L}+(?<w>(\p{L})(?<=\1\P{L}+\1)\p{L}*))+\b";
var result = Regex.Match(text, pattern, RegexOptions.IgnoreCase)?.Groups["w"].Captures
.Cast<Capture>()
.Select(x => x.Value);
Console.WriteLine(string.Join(", ", result)); // => word, duel, Limes, said
A C# demo version without using LINQ:
string text = "One word, duel. Limes said bye.";
string pattern = @"\b(?<w>\p{L}+)(?:\P{L}+(?<w>(\p{L})(?<=\1\P{L}+\1)\p{L}*))+\b";
Match result = Regex.Match(text, pattern, RegexOptions.IgnoreCase);
List<string> output = new List<string>();
if (result.Success)
{
foreach (Capture c in result.Groups["w"].Captures)
output.Add(c.Value);
}
Console.WriteLine(string.Join(", ", output));