You can alternate formatting on a String with a ForEach
loop wrapped in an HStack
, although that comes with its own issues. The following code will let you conditionally apply formatting based on the word's location in the string:
struct FancyTextView: View {
let label: String
var splicedLabel: [String] {
return label.split(separator: " ").map(String.init)
}
var body: some View {
HStack {
ForEach(0..<splicedLabel.count, id: \.self) { index in
Text(self.splicedLabel[index])
.fontWeight(index.isMultiple(of: 2) ? .bold : .regular)
.foregroundColor(index.isMultiple(of: 2) ? .blue : .red)
}
}
}
}
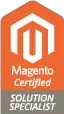
Unfortunately, this simple solution doesn't work with longer strings:

I thought recursion might be useful, but I couldn't think of a way to use recursion with SwiftUI's Text() + Text()
syntax.
Still, you could modify the above code to only format the first word with a larger font and then you could use the Text() + Text()
syntax:

This also removes the unpleasant formatting of the HStack
and ForEach
combination with text. Just change the body to the following:
Text(splicedLabel[0] + " ")
.font(.title)
.fontWeight(.bold)
+ Text(splicedLabel[1])
If you use this example, make sure you change the maxSplits
parameter in .split()
to 1
and check to make sure that there are at least two strings in the resulting array.