The idea is to first identify the text in AlertDialog
by a unique key, tell the driver about it and then perform tap
action on it. In your main code, add key
property to parent widget that has the Text
you want to tap on, for example: I am showing a simple AlertDialog
that show 2 options, as below:
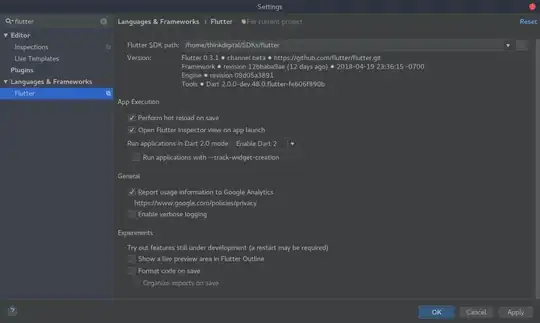
The code for this is:
showDialog(context: context,
builder: (BuildContext context) {
return AlertDialog(
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
GestureDetector(
key: Key('firstOptionKey'),
child: Text('Take a picture'),
onTap: () {},
),
Padding(
padding: EdgeInsets.all(8.0),
),
GestureDetector(
key: Key('secondOptionKey'),
child: Text('Select from gallery'),
onTap: () {},
),
],
),
),
);
});
As you can see, I've wrapped both the text with GestureDetector
that allows us to tap on them. Also, I used key
property for each GestureDetector
to uniquely identify both texts.
In your driver test, you then simply need to identify each text using byValueKey
and tell driver to tap on it, as below:
test('test dialog', () async {
final firstText = find.byValueKey('firstOptionKey');
await driver.waitFor(find.text('Tap'));
await driver.tap(find.text('Tap')); // tap on this button to open alertDialog
await driver.waitFor(firstText); // wait for flutter driver to locate and identify the element on screen
await driver.tap(firstText);
print('tapped on it');
});

Hope this answers your question.