A common strategy to solve complex computing tasks, is to break them into small, well defined manageable tasks. Divide and conquer.
This also applies to gui: break the design into small, easy to layout containers.
In this case, consider dividing the design into 4 areas (JPanels) nested in a main JPanel:
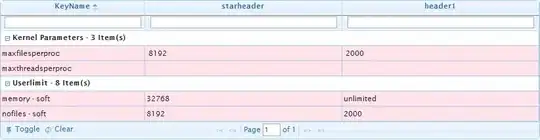
This basic layout, that can get you started, can be achieved like so:
import java.awt.Color;
import java.awt.Dimension;
import javax.swing.BoxLayout;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Gui {
private final String text ="Growing ";
private JPanel grid;
private JFrame f;
Gui() {
creategui();
}
void creategui(){
f = new JFrame("Hexagon Cross For Less");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setLocationRelativeTo(null);
f.setLayout(new BoxLayout(f.getContentPane(),BoxLayout.Y_AXIS));
JPanel primes = new JPanel();
primes.setPreferredSize(new Dimension(1000,120));
primes.setBackground(Color.CYAN);
f.add(primes);
JPanel xFiles = new JPanel();
xFiles.setPreferredSize(new Dimension(1000,120));
xFiles.setBackground(Color.YELLOW);
f.add(xFiles);
JPanel actions = new JPanel();
actions.setPreferredSize(new Dimension(1000,120));
actions.setBackground(Color.GREEN);
f.add(actions);
JPanel status = new JPanel();
status.setPreferredSize(new Dimension(1000,40));
status.setBackground(Color.LIGHT_GRAY);
f.add(status);
f.pack();
f.setVisible(true);
}
public static void main(String[] args) {
new Gui();
}
}
it produces the following layout:
It can be better structured by representing each such distinct area (JPanel
) in a separate class.
The following code produces exactly the same layout (it is a single-file mre. Copy past it to Gui.java
and run):
import java.awt.Color;
import java.awt.Dimension;
import javax.swing.BoxLayout;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Gui {
private final String text ="Growing ";
private JPanel grid;
private JFrame f;
Gui() {
creategui();
}
void creategui(){
f = new JFrame("Hexagon Cross For Less");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setLocationRelativeTo(null);
f.add(new MainPane());
f.pack();
f.setVisible(true);
}
public static void main(String[] args) {
new Gui();
}
}
class MainPane extends JPanel{
MainPane() {
setLayout(new BoxLayout(this,BoxLayout.Y_AXIS));
add(new Primes());
add(new CrossFiles());
add(new Actions());
add(new Status());
}
}
class Primes extends JPanel{
Primes() {
setPreferredSize(new Dimension(1000,120));
setBackground(Color.CYAN);
}
}
class CrossFiles extends JPanel{
CrossFiles() {
setPreferredSize(new Dimension(1000,120));
setBackground(Color.YELLOW);
}
}
class Actions extends JPanel{
Actions() {
setPreferredSize(new Dimension(1000,120));
setBackground(Color.GREEN);
}
}
class Status extends JPanel{
Status() {
setPreferredSize(new Dimension(1000,40));
setBackground(Color.LIGHT_GRAY);
}
}
To continue, apply the same technique and define the layout of each of the 4 sub panels.
The design of Actions
could be :
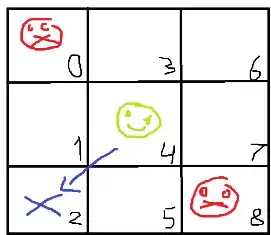
Hint: Primes
and CrossFiles
have exactly the same layout.