You get several issues in your code. Basically, your error is to try to apply straight a code to any dataframe without checking that the dataframe as the same structure as the one used in the example.
Let me explain, here you see the output of the head
of both your data you provided as csv
that I import using this code:
data <- read.table("../Ants.csv", sep = ";", header = T)
data2 <- read.table("../Ant_data_R.csv", sep = ";", header = T)
For Ants
:
> head(data[1:6])
No Sp.1 Sp.2 Sp.3 Sp.4 Sp.5
1 Transect 1 0 0 0 0 0
2 Transect 2 0 0 0 0 0
3 Transect 3 0 4 0 0 2
4 Transect 4 0 0 0 0 0
5 Transect 5 0 1 0 0 1
6 Transect 6 0 1 0 0 0
For Ant_data_R
:
> head(data2[1:6])
Site Habitat No Sp.1 Sp.2 Sp.3
1 CA H 1 NA NA NA
2 CA H 2 NA NA NA
3 CA H 3 NA NA NA
4 CA H 4 NA NA NA
5 CA H 5 NA NA NA
6 CA H 6 NA NA NA
As you can see, 1) there is more descriptive columns in the first columns of the second file. 2) In the second file, missing values are filled with NA
instead of 0
.
So, in your code you can't use straight ants <- data[2:16]
as provided by the example because you will have different output:
Here for the first dataframe:
ants <- data[2:16]
> head(ants[1:6])
Sp.1 Sp.2 Sp.3 Sp.4 Sp.5 Sp.6
1 0 0 0 0 0 15
2 0 0 0 0 0 3
3 0 4 0 0 2 13
4 0 0 0 0 0 15
5 0 1 0 0 1 6
6 0 1 0 0 0 9
And here for the second one:
ants2 <- data2[2:16]
> head(ants2[1:6])
Habitat No Sp.1 Sp.2 Sp.3 Sp.4
1 H 1 NA NA NA NA
2 H 2 NA NA NA NA
3 H 3 NA NA NA NA
4 H 4 NA NA NA NA
5 H 5 NA NA NA NA
6 H 6 NA NA NA NA
As you can see the output is very different. In the first dataframe, it will select numerical values compatibles with the specaccum
function but in the second case you will have character values and NA.
So, the solution is to filter and select only columns of interest. We can do that using dplyr
(other ways exists):
library(vegan)
library(tidyverse)
ants2 <- data2 %>% filter(., Site == "CA") %>% select(., contains("Sp.")) %>% replace(is.na(.),0)
Now the output of ants2
look like:
> head(ants2[1:6])
Sp.1 Sp.2 Sp.3 Sp.4 Sp.5 Sp.6
1 0 0 0 0 0 0
2 0 0 0 0 0 0
3 0 0 0 0 0 0
4 0 0 0 0 0 0
5 0 0 0 0 0 0
6 0 0 0 0 0 0
And if you are applying the specaccum
function and you are plotting them, you get:
Curve = specaccum(ants, method = "random",permutations = 999)
Curve2 = specaccum(ants2, method = "random",permutations = 999)
plot(Curve,ci=0,ci.type=c("line"),col="red",ylab="Number of Species",main="Calitzdorp", ylim = c(2,15))
axis(1, at=1:10)
lines(Curve2,ci=0,ci.type=c("line"),col="blue")
legend("topleft", legend = c("Ants", "Ants_data_R"), col = c("red","blue"), bty = F, lty = 1)
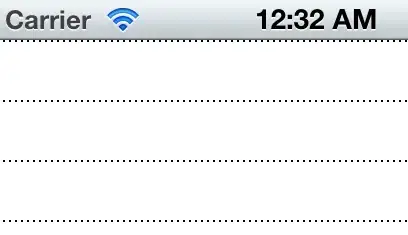
Hope it helps you to figure out your error in the processing of the second dataframe.
Let me know if it is what you were looking for.