From the comments, it seems that you don't want to be limited to detecting whether the mouse moves in the program, and you want to call that beep when the mouse stops moving.
So you can do it by setting the timer and hook.
An application installs the hook procedure by specifying the
WH_MOUSE_LL hook type and a pointer to the hook procedure in a call to
the SetWindowsHookEx function.
If you are unfamiliar with using hooks.Don't worry. There are special documents to explain how to use hooks.
Don't forget to use GetLastInputInfo which can retrieve the time of the last input event.
Please note: This GetLastInputInfo function takes into consideration ALL input events, not just mouse events.
How to use GetLastInputInfo
? Refer @Roger Rowland's answer.
For details, please see my code.
#include <Windows.h>
#include <iostream>
#pragma comment(lib,"Winmm.lib")
using namespace std;
HHOOK mouseHook;
static DWORD t1;
DWORD GetIdleTime()
{
LASTINPUTINFO pInput;
pInput.cbSize = sizeof(LASTINPUTINFO);
if (!GetLastInputInfo(&pInput))
{
// report error, etc.
}
return pInput.dwTime;
}
void CALLBACK f(HWND hwnd, UINT uMsg, UINT timerId, DWORD dwTime)
{
DWORD t2 = GetIdleTime();
if (t2 != t1) //When the mouse is moving, t1 == t2
{
MessageBeep(MB_ICONERROR);
}
cout << "t1: " << t1 << endl; //For test
cout << "t2: " << t2 << endl;
cout << endl;
}
LRESULT __stdcall MouseHookCallback(int nCode, WPARAM wParam, LPARAM lParam)
{
if (nCode >= 0)
{
Sleep(10); //Used to correct the value of t1
t1 = GetIdleTime();
}
return CallNextHookEx(mouseHook, nCode, wParam, lParam);
}
void SetHook()
{
if (!(mouseHook = SetWindowsHookEx(WH_MOUSE_LL, MouseHookCallback, NULL, 0)))
{
cout << "Failed to install mouse hook!" << endl;
}
}
void ReleaseHook()
{
UnhookWindowsHookEx(mouseHook);
}
int main()
{
SetHook(); //Install hook
SetTimer(NULL, 0, 3000, (TIMERPROC)&f); //Check every three seconds
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
Debug:
If mouse is moving, t1 == t2.
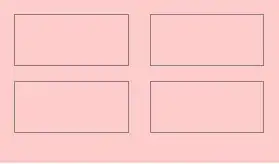
If mouse stop moving, t1 != t2. MessageBeep
will be called.
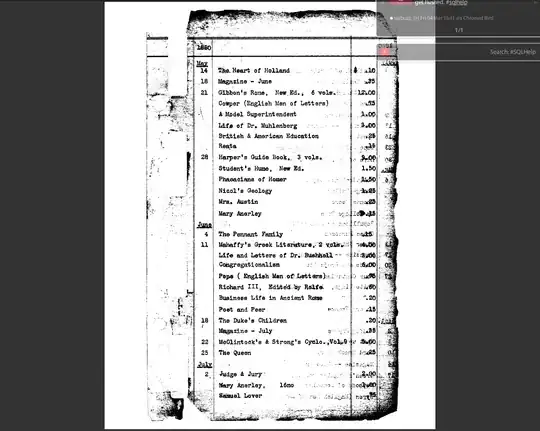
If you don't need a console, please replace int main()
with int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)