You can use the verticalAlignment
parameter in the Row
.
Something like:
Row(
modifier = Modifier.padding(20.dp),
verticalAlignment = Alignment.CenterVertically
) {
Text(text = "Hello!")
Spacer(modifier = Modifier.width(10.dp))
Button(onClick = { /*do something*/ },
modifier = Modifier.padding(25.dp)) {
Text(text = "Click")
}
}
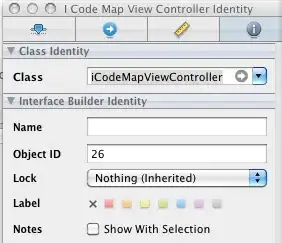
You can also use the align
modifier defined in the RowScope
in each composable in the Row
.
Pls note that:
Align the element vertically within the Row
. This alignment will have priority over the Row
's verticalAlignment
parameter.
Row(
verticalAlignment = Alignment.CenterVertically
) {
// The child with no align modifier is positioned by default so that is in the middle of
// the vertical axis.
Box(Modifier.size(80.dp, 40.dp).background(Teal200))
// Gravity.Top, the child will be positioned so that its top edge is aligned to the top
// of the vertical axis.
Box(Modifier.size(80.dp, 40.dp).align(Alignment.Top).background(Color.Red))
// Gravity.Center, the child will be positioned so that its center is in the middle of
// the vertical axis.
Box(Modifier.size(80.dp, 40.dp).align(Alignment.CenterVertically).background(Color.Yellow))
// Gravity.Bottom, the child will be positioned so that its bottom edge is aligned to the
// bottom of the vertical axis.
Box(Modifier.size(80.dp, 40.dp).align(Alignment.Bottom).background(Color.Green))
}
