You can use pyplot.bar
to make a bar plot. You can check the documentation for more details about it, but you need the location of the bars (in your case, the value_x_mean
variables) and the height of the bars (value_x_count
). I suggest renaming your parameters inside the dictionary to make iterating through it easier:
data = {
"value-A" : {
"value-a-mean" : 10.0,
"value-a-count" : 100
},
"value-B" : {
"value-b-mean" : 2.0,
"value-b-count" : 150
},
"value-C" : {
"value-c-mean" : 6.6,
"value-c-count" : 220
},
"value-D" : {
"value-d-mean" : 11.4,
"value-d-count" : 200
}
}
for key, value in data.items():
for sub_key, sub_value in value.items():
# When you do 'value_a_mean'.split('-') you get ['value', 'a', 'mean']
# We take the last item from this list [-1], so just 'mean' or 'count'
value[sub_key.split('-')[-1]] = value[sub_key]
del value[sub_key]
Now data
will look like this:
{
"value-A" : {
"mean" : 10.0,
"count" : 100
},
"value-B" : {
"mean" : 2.0,
"count" : 150
},
"value-C" : {
"mean" : 6.6,
"count" : 220
},
"value-D" : {
"mean" : 11.4,
"count" : 200
}
}
Then you can do:
from matplotlib import pyplot
data = {
"value-A" : {
"mean" : 10.0,
"count" : 100
},
"value-B" : {
"mean" : 2.0,
"count" : 150
},
"value-C" : {
"mean" : 6.6,
"count" : 220
},
"value-D" : {
"mean" : 11.4,
"count" : 200
}
}
means, counts, labels = [], [], []
# Read the dictionary and save values
for key, value in data.items():
labels.append(key)
means.append(value['mean'])
counts.append(value['count'])
# Bar plot
pyplot.bar(means, counts, tick_label=labels)
pyplot.show()
Result:
You can do this for as many other dictionaries as you want and the bar plots will show up together. You can use a legend to label them:
# Random 2nd dictionary
data2 = {
"value-A" : {
"mean" : 20.0,
"count" : 100
},
"value-B" : {
"mean" : 3.0,
"count" : 150
},
"value-C" : {
"mean" : 5.6,
"count" : 220
},
"value-D" : {
"mean" : 8.4,
"count" : 200
}
}
means, counts, labels = [], [], []
for key, value in data.items():
means.append(value['mean'])
counts.append(value['count'])
pyplot.bar(means, counts, label="Dataset 1")
means, counts, labels = [], [], []
for key, value in data2.items():
means.append(value['mean'])
counts.append(value['count'])
pyplot.bar(means, counts, label="Dataset 2")
pyplot.legend()
pyplot.show()
Result:
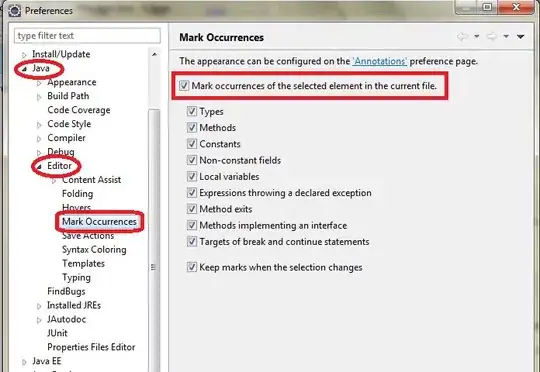