You can also use one of the overloaded methods of [System.Windows.Forms.MessageBox]::Show()
which allows you to add the owner window in order to have the messagebox be topmost to that.
By using $null
there, your messagebox will be topmost to all opened windows:
function Show-MessageBox {
[CmdletBinding()]
Param (
[Parameter(Mandatory = $false)]
[string]$Title = 'MessageBox in PowerShell',
[Parameter(Mandatory = $true)]
[string]$Message,
[Parameter(Mandatory = $false)]
[ValidateSet('OK', 'OKCancel', 'AbortRetryIgnore', 'YesNoCancel', 'YesNo', 'RetryCancel')]
[string]$Buttons = 'OKCancel',
[Parameter(Mandatory = $false)]
[ValidateSet('Error', 'Warning', 'Information', 'None', 'Question')]
[string]$Icon = 'Information',
[Parameter(Mandatory = $false)]
[ValidateRange(1,3)]
[int]$DefaultButton = 1
)
# determine the possible default button
if ($Buttons -eq 'OK') {
$Default = 'Button1'
}
elseif (@('AbortRetryIgnore', 'YesNoCancel') -contains $Buttons) {
$Default = 'Button{0}' -f [math]::Max([math]::Min($DefaultButton, 3), 1)
}
else {
$Default = 'Button{0}' -f [math]::Max([math]::Min($DefaultButton, 2), 1)
}
Add-Type -AssemblyName System.Windows.Forms
# added from tip by [Ste](https://stackoverflow.com/users/8262102/ste) so the
# button gets highlighted when the mouse hovers over it.
[void][System.Windows.Forms.Application]::EnableVisualStyles()
# Setting the first parameter 'owner' to $null lets he messagebox become topmost
[System.Windows.Forms.MessageBox]::Show($null, $Message, $Title,
[Windows.Forms.MessageBoxButtons]::$Buttons,
[Windows.Forms.MessageBoxIcon]::$Icon,
[Windows.Forms.MessageBoxDefaultButton]::$Default)
}
With this function in place, you call it like:
Show-MessageBox -Title 'Important message' -Message 'Hi there!' -Icon Information -Buttons OK

Edit
As asked by Ste, the above function shows the messagebox TopMost
. That however does not mean it is Modal
. It only means the box is shown on top when first displayed, but can be pushed to the background by activating other windows.
For a real Modal messagebox that cannot be pushed to the background, I use this:
function Show-MessageBox {
[CmdletBinding()]
param(
[parameter(Mandatory = $true, Position = 0)]
[string]$Message,
[parameter(Mandatory = $false)]
[string]$Title = 'MessageBox in PowerShell',
[ValidateSet("OKOnly", "OKCancel", "AbortRetryIgnore", "YesNoCancel", "YesNo", "RetryCancel")]
[string]$Buttons = "OKCancel",
[ValidateSet("Critical", "Question", "Exclamation", "Information")]
[string]$Icon = "Information"
)
Add-Type -AssemblyName Microsoft.VisualBasic
[Microsoft.VisualBasic.Interaction]::MsgBox($Message, "$Buttons,SystemModal,$Icon", $Title)
}
Show-MessageBox -Title 'Important message' -Message 'Hi there!' -Icon Information -Buttons OKOnly
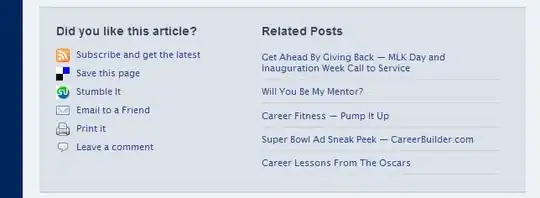