After many attempts I made a solution. I assume that you have a padding outside a radius (for instance, if you have radius = 10000 m, then it will be 2500 m left and right). Also you should have an accuracy in meters. You can set suitable zoom with recoursion (binary search). If you change moveCamera
to animateCamera
, you will get an interesting animation of search. The larger radius, the more accurate zoom value you will receive. This is a usual binary search.
private fun getCircleZoomValue(latitude: Double, longitude: Double, radius: Double,
minZoom: Float, maxZoom: Float): Float {
val position = LatLng(latitude, longitude)
val currZoom = (minZoom + maxZoom) / 2
val camera = CameraUpdateFactory.newLatLngZoom(position, currZoom)
googleMap!!.moveCamera(camera)
val results = FloatArray(1)
val topLeft = googleMap!!.projection.visibleRegion.farLeft
val topRight = googleMap!!.projection.visibleRegion.farRight
Location.distanceBetween(topLeft.latitude, topLeft.longitude, topRight.latitude,
topRight.longitude, results)
// Difference between visible width in meters and 2.5 * radius.
val delta = results[0] - 2.5 * radius
val accuracy = 10 // 10 meters.
return when {
delta < -accuracy -> getCircleZoomValue(latitude, longitude, radius, minZoom,
currZoom)
delta > accuracy -> getCircleZoomValue(latitude, longitude, radius, currZoom,
maxZoom)
else -> currZoom
}
}
Usage:
if (googleMap != null) {
val zoom = getCircleZoomValue(latitude, longitude, radius, googleMap!!.minZoomLevel,
googleMap!!.maxZoomLevel)
}
You should call this method not earlier than inside first event of googleMap?.setOnCameraIdleListener
, see animateCamera works and moveCamera doesn't for GoogleMap - Android. If you call it right after onMapReady
, you will have a wrong distance, because the map will not draw itself that time.
Warning! Zoom level depends on location (latitude). So that the circle will have different sizes with the same zoom level depending on distance from equator (see Determine a reasonable zoom level for Google Maps given location accuracy).
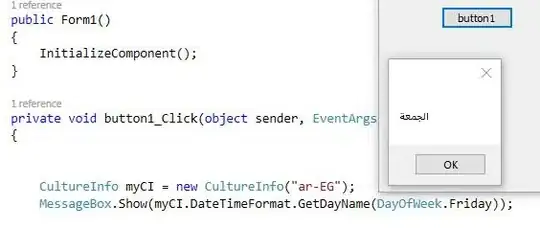