Here's one way of doing it...
#!/usr/bin/env python3
import numpy as np
import cv2
# Open the image and make black version, same size, to fill randomly
im = cv2.imread('paddington.png')
fade = np.zeros_like(im)
# Generate a randomly shuffled array of the coordinates
X,Y = np.where(im[...,0]>=0)
coords = np.column_stack((X,Y))
np.random.shuffle(coords)
for n, c in enumerate(list(coords)):
# Copy one original pixel across to image we are fading in
x, y = c
fade[x,y] = im[x,y]
# It takes 1ms to update the image, so we don't update after every pixel
if n % 500 == 0:
cv2.imshow('Fading in...', fade)
cv2.waitKey(1)
# Image should now be completely faded in
cv2.imshow('Fading in...', fade)
cv2.waitKey()
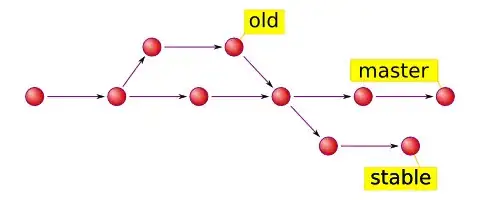
Keywords: Python, OpenCV, fade, fade in, fade from black, fade from white, image processing, video.