User agent:
You can get it via JavaScript interop. Follow this easy steps:
On your _Host.cshtml
file:
<script>
window.getUserAgent = () => {
return navigator.userAgent;
};
</script>
To get user agent on any Blazor page:
var remoteUserAgent = await JSRuntime.InvokeAsync<string>("getUserAgent");
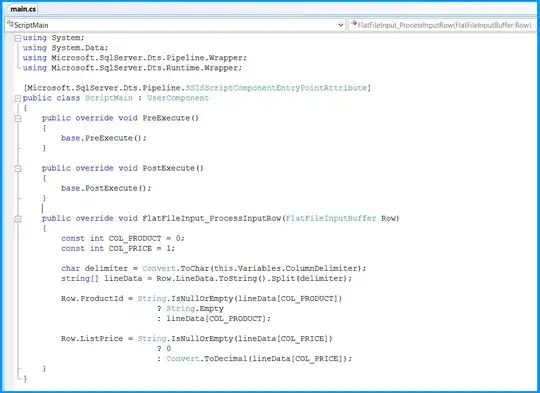
Notice you don't need to send user agent on each request, you can send it with your first request, all other client-side communication will be through the same socket.
Remote IP:
Bad news: "There is no a good way to do this at the moment. We will look into how we can provide make this information available to the client." more info at How do I get client IP and browser info in Blazor?
Edited Dec 31, 2019:
I guess I overthink about how to access to HttpContext
. Reading some @enet comments and "How to use the HttpContext object in server-side Blazor to retrieve information about the user, user agent" post, I realize that you can access HttpContext
via first request and not via SignalR requests. I mean, Blazor Server sends the App to client (browser) via Http Request, at this moment, when Blazor server app is served to client, you have access to HttpContext
. I copy-paste Michael Washington's answer here (now is removed), this answer is very closed to Soroush Asadi's comment:
In your startup file add to ConfigureServices(IServiceCollection services)
:
services.AddHttpContextAccessor();
In a .razor
page add:
@using Microsoft.AspNetCore.Http
@inject IHttpContextAccessor httpContextAccessor
//Then call:
httpContextAccessor.HttpContext.Connection?.RemoteIpAddress.ToString();