(Using Java syntax rather than Scala)
tl;dr
a specific timezone to which I know it corresponds (GMT-5)
GMT-5
is not a time zone, it is a mere offset. A time zone has a name in Continent/Region
format, such as Africa/Tunis
. A time zone is a history of changes in offset used by a region.
You cannot reliably determine a time zone from an offset. Many time zones may share the same offset on a particular moment, but at other moments may differ. So you would only be making a wild guess. Apply a time zone only if you know the particular zone with certainty.
Instead, use the offset itself to determine a moment, without a zone. Here is a one-line solution.
LocalDateTime // Represent a date with time-of-day but lacking the context of an offset-from-UTC or a time zone.
.parse( // When parsing, no need to specify a formatting pattern when the input complies with the ISO 8601 standard.
"2019-12-23 13:35:00".replace( " " , "T" ) // Comply with ISO 8601 standard formatting, replacing SPACE in the middle with an uppercase `T`.
) // Returns a `LocalDateTime` object.
.atOffset( // Determine a moment by placing the date and time-of-day of a `LocalDateTime` into the context of an offset-from-UTC.
ZoneOffset.ofHours( -5 ) // Represent an offset-from-UTC with `ZoneOffset`, merely a number of hours-minutes-seconds ahead or behind the prime meridian.
) // Returns a `OffsetDateTime` object.
.toString() // Generate text in standard ISO 8601 format.
Run code live.
2019-12-23T13:35-05:00
java.time
ISO 8601
Your input string is nearly in ISO 8601 standard format. To comply, replace the SPACE in the middle with a T
.
String input = "2019-12-23 13:35:00".replace( " " , "T" ) ;
LocalDateTime
Parse as a LocalDateTime
given your input's lack of a time zone or offset-from-UTC.
LocalDateTime ldt = LocalDateTime.parse( input ) ;
Be aware that a LocalDateTime
is not a moment, is not a point on the timeline. In your example of approximately 1:30 PM, we do not know if this means 1:30 PM in Tokyo Japan or 1:30 PM in Toledo Ohio US, two very different moment many hours apart.
OffsetDateTime
You claim to know for certain that this date and time was meant to be seen through the lens of an offset five hours behind UTC (generally west of the prime meridian).
ZoneOffset offset = ZoneOffset.ofHours( -5 ) ; // Five hours before UTC.
So let's apply that ZoneOffset
to get an OffsetDateTime
.
OffsetDateTime odt = ldt.atOffset( offset ) ;
See this code run live at IdeOne.com.
odt.toString(): 2019-12-23T13:35-05:00
ZonedDateTime
A time zone is always preferable to a mere offset-from-UTC. An offset is a number of hour--minutes-seconds, nothing more. A time zone is much more. A time zone is a history of past, present, and future changes to the offset used by the people of a particular region.
But do not guess at a time zone. Apply a zone only if you know your LocalDateTime
object was originally intended for that zone.
Specify a proper time zone name in the format of Continent/Region
, such as America/Montreal
, Africa/Casablanca
, or Pacific/Auckland
. Never use the 2-4 letter abbreviation such as EST
or IST
as they are not true time zones, not standardized, and not even unique(!).
ZoneId z = ZoneId.of( "America/Panama" ) ;
ZonedDateTime zdt = ldt.atZone( z ) ;
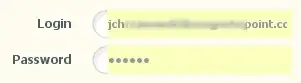
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.