tl;dr
If you want to get the first moment of the day on that date as seen in UTC, transformed into a count of milliseconds since 1970-01-01T00:00Z :
LocalDate // Represent a date, without time-of-day and without time zone.
.of( 2019 , 3 , 4 ) // Determine a date. Uses sane numbering, 1-12 for January-December.
.atStartOfDay( // Determine first moment of the day.
ZoneOffset.UTC // Get the first moment as seen at UTC (an offset of zero hours-minutes-days.
) // Returns a `ZonedDateTime` object.
.toInstant() // Convert from a `ZonedDateTime` to a simple `Instant` object, which is always in UTC, and has methods to get count-from-epoch.
.toEpochMilli() // Get a count of milliseconds since the epoch reference of first moment of 1970 to UTC.
java.time
You are using terrible date-time classes that were supplanted years ago by the modern java.time classes defined in JSR 310.
To get a count of milliseconds or whole seconds since the epoch reference of first moment of 1970 in UTC, 1970-01-01T00:00Z, use Instant
.
long millis = Instant.now().toEpochMilli() ;
Or:
long seconds = Instant.now().getEpochSecond() ;
For a date, you must determine the first moment of the day on that date. This not always the time 00:00, so let java.time determine that moment. Doing so requires a time zone. The day starts earlier in the East than in the west, varying around the globe by time zone.
LocalDate ld = LocalDate.of( 2019 , 3 , 4 ) ;
ZoneId z = ZoneId.of( "Africa/Tunis" ) ;
ZonedDateTime zdt = ld.atStartOfDay( z ) ;
Instant instant = zdt.toInstant() ;
long millis = Instant.now().toEpochMilli() ;
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
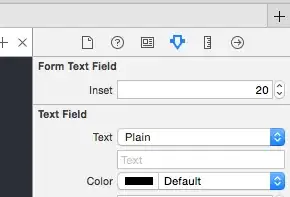