It depends on whether you want to handle the tap in your custom view object or in the view controller.
If in the view, add this to it's init
or other proper place:
UITapGestureRecognizer* tapRecognizer = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(handleTap:)];
[self addGestureRecognizer:tapRecognizer];
[tapRecognizer release];
If in the view controller, add this in the viewDidLoad
(or other proper place):
UITapGestureRecognizer* tapRecognizer = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(handleTap:)];
[yourCustomView addGestureRecognizer:tapRecognizer];
[tapRecognizer release];
handler is the same:
- (void)handleTap:(UITapGestureRecognizer*)recognizer
{
// Do Your thing.
if (recognizer.state == UIGestureRecognizerStateEnded)
{
}
}
Take a look at the SimpleGestureRecognizers example and you should get a pretty good idea.
---- Updated 10/1/2012----
For those of you who like to use storyboard/nib, this is super simple!
Open your storyboard/nib.
Drag-b-drop the kind of recognizer you want from the Object Library onto the UI element you want.
Right-click on the recognizer object, then connect its selector
to an IBAction in the File's Owner (usually an UIViewController.) If you need to, connect the delegate as well.
You're done!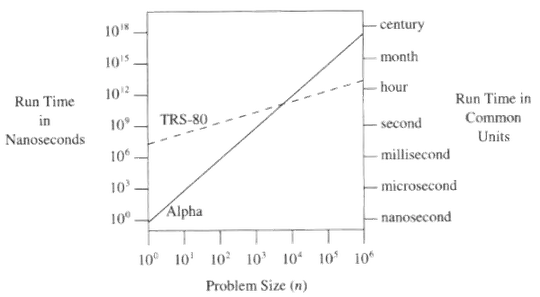