I'm not entirely certain where you want to draw your circle, but I present here three different circle positions, and a simpler fourth alternative just for highlighting a specific date. A demo image is shown at the bottom. First, let's just plot some data:
import matplotlib.pyplot as plt
dates = ['2019-10-31', '2019-11-01', '2019-11-02', '2019-11-03']
y = [i*i for i in range(len(dates))] # some random y values
# An arbitrary date value to encircle
encircled_date = dates[1]
val_of_encircled_date = y[1]
# Plot the graph
fig, ax = plt.subplots()
ax.plot_date(dates,y,'-')
bottom, top = plt.ylim() # Later, we'll need the min value of the y-axis for correct positioning of circle
Now, if you just want a circle at the graph, as it passes through your specific date, the simplest and (imho) best approach is to simply replot that specific value, but with markerstyle='o'
. Adjust marker size, line width and color to your preferences:
# Plot a circle around specific graph value
ax.plot_date(encircled_date, val_of_encircled_date,
'og', # marker style 'o', color 'g'
fillstyle='none', # circle is not filled (with color)
ms=10.0) # size of marker/circle
Then, if you instead wanted a circle around the date-tick, on the x-axis for your specific date, it is a little more tricky depending on what details you need to encircle. Of course, you could use the approach above to get a small circle around the tick only, but I'll show a more advanced approach based on another SO-question:
# Plot a circle around the 'tick' of specific date on the x-axis
circle1 = plt.Circle((encircled_date, bottom), # position
1.0 / len(dates), # radius
color='r',
clip_on=False, # allow drawing outside of axes
fill=False)
ax.add_artist(circle1)
The above solution only encircles the tick, and not the date label itself. We may micro adjust the circle to fit the date-label inside, by tuning two offset parameters,
# Plot a circle around the specific date's label on the x-axis
pos_offset = 0.5
len_offset = 0.4
circle2 = plt.Circle((encircled_date, bottom-pos_offset), # position
(1.0+len_offset) / len(dates), # radius
color='purple',
clip_on=False, # allow drawing outside of axis
fill=False)
ax.add_artist(circle2)
However, this tuning may be a tedious task. If your objective is only to emphasize this particular date, it may be better to simply reconfigure the x-label. You may for instance change the color of the label like this,
ax.get_xticklabels()[2].set_color("red")
ax.get_xticklabels()[2].set_weight("bold")
The four different approaches are shown in the image below. I hope this helps.
One final remark: When you get a densely populated x-axis of dates, it might be worthwhile looking into more advanced formatting of date-labels which you can read all about in the official documentation. For instance, they show how to neatly rotate the labels to fit them closer together without overlapping.
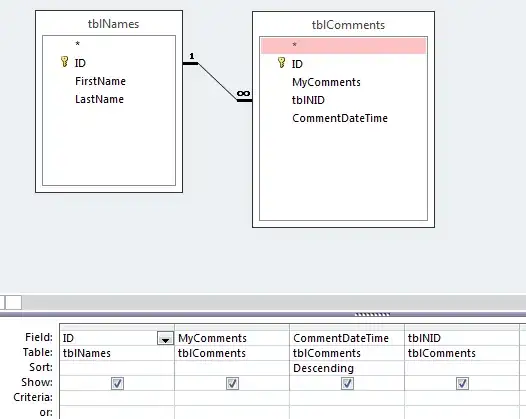