tl;dr
LocalDate // Represent a date-only value without a time-of-day and without a time zone.
.now( // Determine the current date as seen through the wall-clock time used by people in certain region (a time zone).
ZoneId.of( "America/Montreal" ) // Real time zone names have names in the format of `Continent/Region`. Never use 2-4 letter pseudo-zones such as `IST`, `PST`, or `CST`, which are neither standardized nor unique.
) // Return a `LocalDate`.
.with( // Move from one date another by passing a `TemporalAdjuster` implementation.
TemporalAdjusters // Class providing several implementations of `TemporalAdjuster`.
.firstDayOfNextMonth() // This adjuster finds the date of the first of next month, as its name suggests.
) // Returns another `LocalDate` object. The original `LocalDate` object is unaltered.
.toString() // Generate text in standard ISO 8601 format of YYYY-MM-DD.
See this code run live at IdeOne.com.
2020-02-01
Details
You are using terrible date-time classes that were made obsolete years ago by the unanimous adoption of JSR 310 defining the java.time classes.
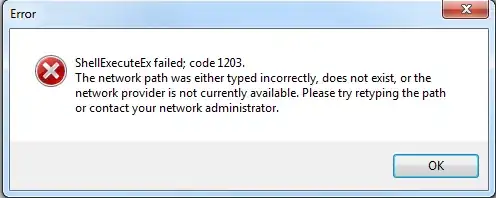
The Answer by deHaar is correct. Here is an even shorter solution.
TemporalAdjuster
To move from one date to another, the java.time classes include the TemporalAdjuster
interface. Pass one of these objects to the with
method found on many of the other java.time classes.
TemporalAdjusters.firstDayOfNextMonth()
Several implementations of that interface are found in the class TemporalAdjusters
(note the s
plural). One of those is firstDayOfNextMonth()
, just what you need.
Get today's date. A time zone is required, as for any given moment the date varies around the globe by time zone. If omitted, your JVM's current default time zone is implicitly applied. Better to be explicit.
ZoneId z = ZoneId.of( "Asia/Tokyo" ) ;
LocalDate today = LocalDate.now( z ) ;
Get your TemporalAdjuster
object.
TemporalAdjuster ta = TemporalAdjusters.firstDayOfNextMonth() ;
Apply that adjuster to get another LocalDate
object. Note that java.time classes are immutable by design. So we get a new object rather than altering the original.
LocalDate firstOfNextMonth = today.with( ta ) ;
We can shorten this code to a one-liner, if desired.
LocalDate firstOfNextMonth =
LocalDate
.now(
ZoneId.of( "Africa/Tunis" )
)
.with(
TemporalAdjusters.firstDayOfNextMonth()
)
;
Text
Your desired output format of YYYY-MM-DD complies with the ISO 8601 standard used by default in the java.time classes when parsing/generating text. So no formatting pattern need be specified.
String output = firstOfNextMonth.toString() ;
2020-02-01
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?

The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.