The idea of calee's solution is finding all Input cells, and then matching the source of code cell.
I enhance his solution in two aspects:
- Works in JupyterLab
- Works for duplicated cells (i.e., have same source content)
The idea is matching the cell id in metadata, which is unique for each cell.
You might need to install ipynbname
since the script needs to get current notebook path in get_notebook_cells
.
pip install ipynbname
Here is the script:
import nbformat
import ipynbname
def get_notebook_cells(notebook_path=None, cell_types=["markdown", "code", "raw"]):
if not notebook_path:
notebook_path = ipynbname.path()
with open(notebook_path, "r", encoding="utf-8") as rf:
nb = nbformat.read(rf, as_version=4)
cells = [cell for i, cell in enumerate(nb.cells) if cell["cell_type"] in cell_types]
return cells
def get_cell_id():
cell_id = get_ipython().get_parent()["metadata"]["cellId"]
return cell_id
def get_cell_index():
cell_id = get_cell_id()
cells = get_notebook_cells()
for idx, cell in enumerate(cells):
if cell["id"] == cell_id:
return idx
print(get_cell_index())
Here is the example of execution:
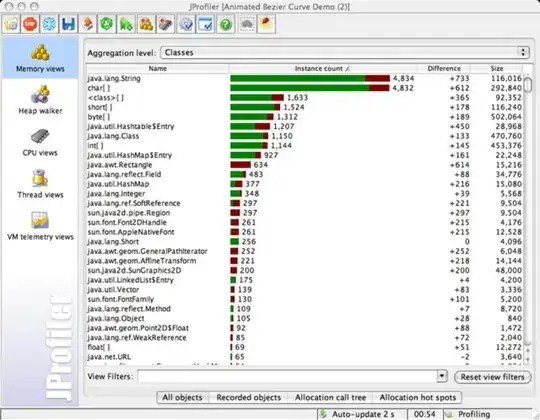
Notes:
- In my case, I put the
get_cell_index()
in another file to make it easily re-used by other notebooks.
- The cell index starts from 0.