You should always aim to capture what exists in reality. A Customer
instance does not have a 1 to 1 relationship with a ProductList
because a ProductList
can be viewed by more than one Customer
at a time, and the Customer
in no way owns that list.
What is probably closer to reality is:
- Every
Supermarket
individual manages one Inventory
individual
- Every
Inventory
individual:
- is managed by one
Supermarket
individual
- comprises
Inventory Item
individuals
- Every
Inventory Item
individual
- is comprised by one
Inventory
individual
- describes
Product
individuals
- Every
Product
individual
- is described by one
Inventory Item
individual
- is located at one
Physical Location
individual
- Every
User Account
individual
- identifies one Person individual
- plays Role individuals
- Every Role individual provides Capability individuals
In real life, people play roles. These roles might be “customer”, “doctor”, or “police officer”. Every individual Role
has a set of capabilities it can perform. In an OO system, every individual Role
can use operations to implement its capabilities, such as purchaseProduct()
, prescribeMedication()
, or writeMovingViolation()
.
There are multiple ways to represent these roles and capabilities in an OO system. In one approach, a customer
instance of a Role
might be configured to allow access to queryInventory()
and purchaseProduct()
operations on the Supermarket
and InventoryItem
classes respectively. An owner
instance of a Role
1 might be configured to allow access to addInventoryItem()
and removeInventoryItem()
operations on the Inventory
class.
Here is an example of a UML model:
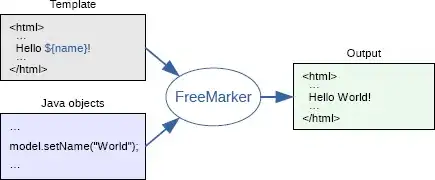
In another approach, you might create singleton subclasses of the Role
class, called CustomerRole
and OwnerRole
, and then have each of those subclasses invoke operations. You might put your viewProducts()
and addProducts()
operations into those singletons.
1 Consider calling this role “manager”, so the owner of the supermarket can hire other people to do the work.