In order to use Windows Forms you need to modify .csproj
:
- set
UseWindowsForms
to true
- append
-windows
to TargetFramework
(e.g. net6.0-windows
)
ConsoleApp.csproj
:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net6.0-windows</TargetFramework>
<UseWindowsForms>true</UseWindowsForms>
</PropertyGroup>
</Project>
Program.cs
:
System.Console.WriteLine("Hello, Console!");
System.Windows.Forms.MessageBox.Show("Hello, Popup!");
Result:
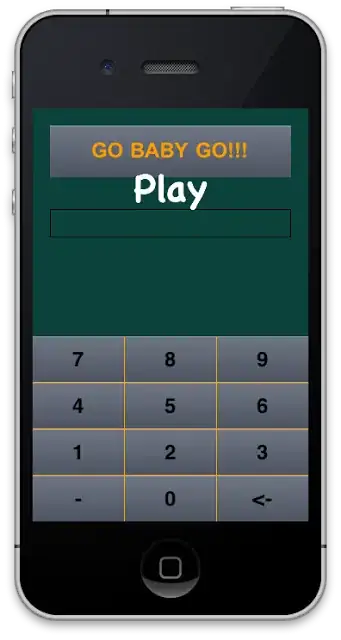
Notes:
- I checked this solution on .NET Core 3.1, .NET 5 and .NET 6 on Windows x64.
- If you don't want console window to be shown, set
OutputType
in .csproj
to WinExe
instead of Exe
.
- Windows Forms works only on Windows, so as this solution.
- On .NET Core 3.1 there is no requirement to change target framework to Windows, however it is still impossible to publish executable for Linux OS.
- If you need cross-platform solution that shows popups on Windows and only use console on Linux – you can create custom build configuration and use preprocessor directives as in the example below.
Cross-platform ConsoleApp.csproj
:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<OutputType>Exe</OutputType>
<TargetFramework>net6.0</TargetFramework>
<Configurations>Debug;Release;WindowsDebug;WindowsRelease</Configurations>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)' == 'WindowsDebug' Or '$(Configuration)' == 'WindowsRelease'">
<DefineConstants>WindowsRuntime</DefineConstants>
<TargetFramework>net6.0-windows</TargetFramework>
<UseWindowsForms>true</UseWindowsForms>
</PropertyGroup>
</Project>
Cross-platform Program.cs
System.Console.WriteLine("Hello, Console!");
#if WindowsRuntime
System.Windows.Forms.MessageBox.Show("Hello, Popup!");
#endif