Objective: To be able to click a Shape, rectangle as per OP's code, and change its color
Steps:
- Draw a rectangle
- We then check if the click event occurs within the bounds of the rect
- OnClick we set the
IsRectClick
flag to true
- Redraw the Panel
EDIT: Added another feature
- Also can now move the shape by dragging
- Redraws shape when click on another area
Form1.cs
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApp2
{
public partial class Form1 : Form
{
bool isRectClick = false;
System.Drawing.SolidBrush newColor;
public Rectangle rect = new Rectangle(200, 100, 50, 50);
public Form1()
{
InitializeComponent();
}
private void panel1_Paint(object sender, PaintEventArgs e)
{
if (isRectClick == false)
{
System.Drawing.SolidBrush fillRed = new System.Drawing.SolidBrush(Color.Red);
e.Graphics.FillRectangle(fillRed, rect);
}
else
{
e.Graphics.FillRectangle(newColor, rect);
}
}
private void Panel1_MouseDown(object sender, MouseEventArgs e)
{
if (this.rect.Contains(e.Location))
{
newColor = new System.Drawing.SolidBrush(Color.Blue); //New Color
isRectClick = true;
panel1.Invalidate();
}
else
{
if (e.Button == MouseButtons.Left)
{
rect = new Rectangle(e.X, e.Y, 50, 50);
panel1.Invalidate();
}
}
}
private void Panel1_MouseMove(object sender, MouseEventArgs e)
{
if (e.Button == MouseButtons.Left)
{
int initialX = 0, initialY = 0;
rect.X = (e.X - 200) + initialX;
rect.Y = (e.Y - 100) + initialY;
panel1.Invalidate();
}
}
}
}
Form1.Designer.cs
using System.Drawing;
namespace WindowsFormsApp2
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.panel1 = new System.Windows.Forms.Panel();
this.panel1.SuspendLayout();
this.SuspendLayout();
//
// panel1
//
this.panel1.Location = new System.Drawing.Point(89, 63);
this.panel1.Name = "panel1";
this.panel1.Size = new System.Drawing.Size(646, 342);
this.panel1.TabIndex = 0;
this.panel1.Paint += new System.Windows.Forms.PaintEventHandler(this.panel1_Paint);
this.panel1.MouseDown += new System.Windows.Forms.MouseEventHandler(this.Panel1_MouseDown);
this.panel1.MouseMove += new System.Windows.Forms.MouseEventHandler(this.Panel1_MouseMove);
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 16F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(800, 450);
this.Controls.Add(this.panel1);
this.Name = "Form1";
this.Text = "Form1";
this.panel1.ResumeLayout(false);
this.ResumeLayout(false);
}
#endregion
private System.Windows.Forms.Panel panel1;
}
}
Output
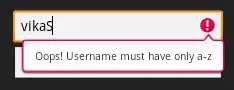