You are on the right track, but are working a bit too hard...
The thing to keep in mind about UI elements in WPF is that they work in layers. Think of elements as a composite of smaller items that you want to stack in a 3-D space. You aren't just working in a 2 dimensional drawing space anymore like a GDI rendering space of WinForms.
So lets logically think about what you want to do here. Lets try to achieve several goals in our solution:
- Lets make a control template that will allow us to reuse our newly minted control.
Lets make the control completely portable in 3 dimensional space i.e. no use of raster images that will distort if we resize our control - lets stick to geometry only for drawing.
Lets make it easy to decide how large to make the triangle in the corner.
Lets decide to allow easy modifications for later, such as triangle placement (you want to change its location), hit testing (should it be part of the text surface or the border?), color (again, border or text?), etc.
Ok, to solve this, I alluded to the idea of objects in layers. Lets divide our drawing surface into a grid:
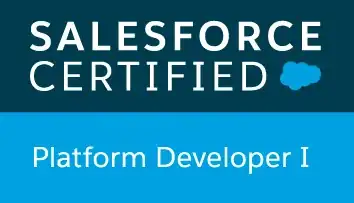
That means we want to use a Grid
control. We want to define two rows and two columns, and we want to TextBox
to cover all 4 cells. We will make sure to set the Rowspan
and Columnspan
to fill the grid.
We will then add a 2nd item, our Polyline
triangle to row 1 column 1, and add it after the text box. That way it's Z-order will be on top.
Here then is the control template:
<Window.Resources>
<Style x:Key="TriangleTextBox" TargetType="{x:Type TextBox}">
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="{x:Type TextBox}">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="10"/>
<RowDefinition Height="6*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="6*" />
<ColumnDefinition Width="10" />
</Grid.ColumnDefinitions>
<TextBox Grid.Row="0" Grid.Column="0" Grid.RowSpan="2" Grid.ColumnSpan="2" Margin="0,0,0,0" Text="{TemplateBinding Text}" BorderThickness="1"/>
<Polygon Grid.Row="0" Grid.Column="1" Points="0,0 10,0 0,10 0,0" Fill="Black" FlowDirection="RightToLeft"/>
</Grid>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
</Window.Resources>
Note that we set the key to "TriangleTextBox".
Now its just a simple matter of using our control template as follows:
<Grid>
<TextBox Style="{StaticResource ResourceKey=TriangleTextBox}" Width="100" Height="20"/>
</Grid>
And there you have it:
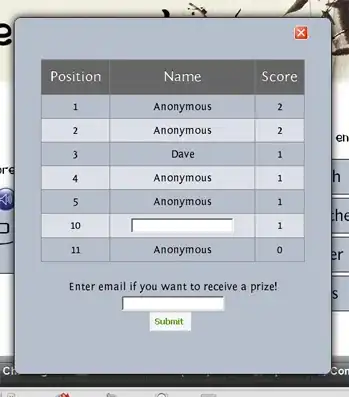
You can expand on this from there.