I found that a content type header really makes it work in chrome after few trial and errors.
But i don't know why chrome does not flush otherwise.
after searching for more answers i read that chrome flushes as you expect only when a valid content type is set. fine.
Here is the code i experimented.
<?php
header('Content-Type: text/html; charset=UTF-8');
echo 'starting...';
flush();
echo 'to sleep...';
flush();
sleep(5);
echo 'awake';
if i do not include the content type header i get like the following in one shot after 5 seconds. so what we expect did not work.
starting...to sleep...awake is displayed and the script terminates.
where as when i gave the content type like the above with the subtype(charset) then
starting...to sleep... is displayed immediately and then after 5 seconds awake is displayed.
i am just blindly assuming that with respect to the content type header chrome shows the output.
Besides when i gave 'Content-Type: text/plain' or 'Content-Type: text/html' it did not work. it worked only with the subtype 'charset=[sometexthere]'.
were as application/json worked. and i did not experiment with more mimes.
The reason i am here is
i wanted to use readystate 3 in ajax response. it works fine except chrome and safari. since chrome is using webkit it is the same in both i think.
in other browsers including IE the flushing is working as expected and also the readystate=3 but in chrome and safari i just used the above workaround.
here is the screenshot of the readystate - responsetext from the above php script
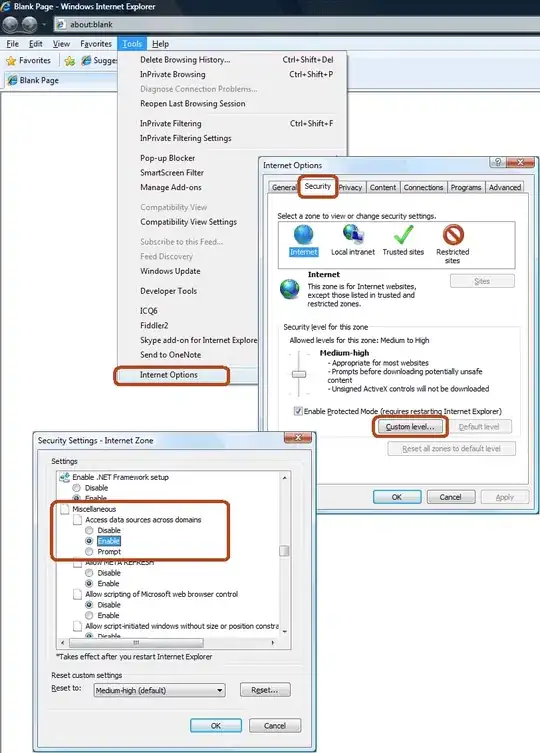
in the image there a two sets of response the first one with readystate 3 and responsetext as empty when content type is not used.
in the second response you can see ready state 3 has responsetext with the expected output. this is when content type is used.
so... Chrome only knows.
when used str_pad
When you use string padding you can get more expected result. i tried with 1024 as the above answers suggested but only with content type set.
if padding is used and no content type is set then it did not work.
and
i had raised a question similar to this and i am going to add my own answer by linking this answer to that and back to back... so that it will be easy for users to get more details. hhmmm.