tl;dr
- Use only java.time classes.
- Never use date-time classes in the
java.util
and java.sql
packages.
You asked:
can we use java.util to save in the database. if so then why java.sql introduced
Use neither. Both are now outmoded by the java.time classes.
And you asked:
while inserting into database should we convert the java.util to java.sql and then save. if so then what will be benefits of it
Use neither. Use only the java.time classes.
myPreparedStatement.setObject( … , myOffsetDatetime ) ;
…and:
OffsetDateTime myOffsetDateTime = myResultSet.getObject( … , OffsetDateTime.class ) ;
JSR 310
You asked:
In java why so much. date and time handling features
The date-time classes in the java.util
and java.sql
packages are terribly flawed in their design. They were built decades ago by people who did not understand the complexities and subtleties of date-time handling.
As a whole the information technology industry largely ignored mastering the details of date-time handling in programming. Quite astounding that so many decades passed with no real complete well-designed solution.
In 2014, the JCP community, including Sun/Oracle, voted unanimously to adopt JSR 310. The java.time classes became part of Java 8 and later. This framework is now leading the industry.
Adopting JSR 310 meant that the date-time classes in the java.util and java.sql packages became legacy, supplanted entirely by the java.time classes. At one point the legacy classes were marked for deprecation, then undone for reasons undisclosed.
Converting
No need for you to ever use those terrible legacy classes again. Avoid java.util.Date
, Calendar
, GregorianCalendar
, SimpleDateFormat
, java.sql.Date
, and so on.
If interoperating with old code not yet updated for java.time, you can convert. Call the new to…
and from…
methods added to the old classes.
Back-ports
For Java 6 & 7, use the back-port library, ThreeTen-Backport. Further adapted for early Android (before 26) in the ThreeTeABP library.
JDBC
JDBC 4.2 and later requires support for exchanging java.time objects with the database. Your JDBC driver has likely been updated by now.
Learn about methods such as PreparedStatement::setObject
and ResultSet::getObject
.
For a moment, that is, for columns of a data type akin to the SQL-standard type TIMESTAMP WITH TIME ZONE
, support for java.time.OffsetDateTime
is required. Oddly, support for the more commonly used Instant
and ZonedDateTime
is not addressed in the JDBC specification. Your driver may or may not support those types. If not, you can easily convert by calling the at…
, to…
, and with…
methods. To make your JDBC code more portable across drivers, exchange only OffsetDateTime
objects, and convert to/from Instant
/ZonedDateTime
as needed.
OffsetDateTime odt = myResultSet.getObject( … , OffsetDateTime ) ;
ZoneId z = ZoneId.of( "Africa/Casablanca" ) ;
ZonedDate zdt = odt.atZoneSameInstant( z ) ;
For columns with only a date and a time-of-day but lacking the context of a time zone or an offset-from-UTC (therefore not a moment), that is, for a data-type akin to the SQL-standard TIMESTAMP WITHOUT TIME ZONE
, use java.time.LocalDateTime
.
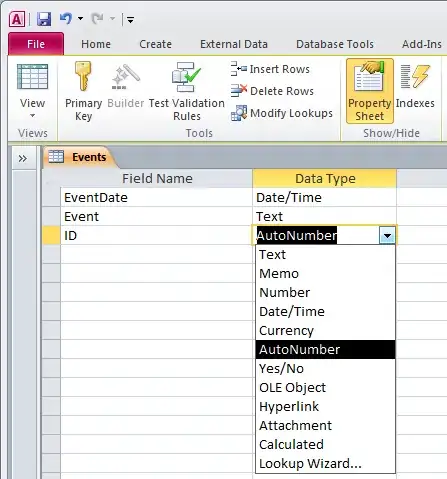
Evolution
The Joda-Time project is the predecessor to java.time. Lessons learned there were used in designing java.time. Joda-Time is now in maintenance-mode.
Joda-Time, JSR 310, java.time, ThreeTen-Backport, and ThreeTen-Extra are all led by the same man, Stephen Colebourne.