Assign each of your plots to a variable like g
, and use plt.close(g.fig)
to remove your unwanted subplots. Or iterate over all sns.axisgrid.FacetGrid
type variables and close them like so:
for p in plots_names:
plt.close(vars()[p].fig)
The complete snippet below does just that. Note that I'm loading the titanic dataset using train_df = sns.load_dataset("titanic")
. Here, all column names are lower case unlike in your example. I've also removed the palette=col_pal
argument since col_pal
is not defined in your snippet.
Plot:
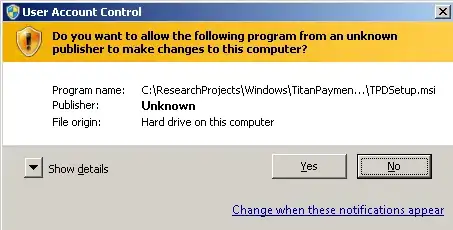
Code:
import seaborn as sns
import matplotlib.pyplot as plt
plt.rcParams['figure.figsize'] = [12, 8]
fig, axes = plt.subplots(nrows=3, ncols=2)
plt.tight_layout()
train_df = sns.load_dataset("titanic")
g = sns.catplot(x='pclass', y='age', data=train_df, kind='box', height=8, ax=axes[0, 0])
h = sns.catplot(x='embarked', y='age', data=train_df, kind='box', height=8, ax=axes[0, 1])
i = sns.catplot(x='sex', y='age', data=train_df, kind='box', height=8, ax=axes[1, 0])
j = sns.catplot(x='sex', y='age', hue='pclass', data=train_df, kind='box', height=8, ax=axes[1, 1])
k = sns.catplot(x='sibsp', y='age', data=train_df, kind='box', height=8, ax=axes[2, 0])
l = sns.catplot(x='parch', y='age', data=train_df, kind='box', height=8, ax=axes[2, 1])
# iterate over plots and run
# plt.close() to prevent duplicate
# subplot setup
var_dict = vars().copy()
var_keys = var_dict.keys()
plots_names = [x for x in var_keys if isinstance(var_dict[x], sns.axisgrid.FacetGrid)]
for p in plots_names:
plt.close(vars()[p].fig)
Please note that you will have to assign your plots to variable names for this to work. If you just add the snippet that closes the plots to the end of your original snippet, the duplicate subplot setup will remain untouched.
Code 2:
import seaborn as sns
import matplotlib.pyplot as plt
plt.rcParams['figure.figsize'] = [12, 8]
fig, axes = plt.subplots(nrows=3, ncols=2)
plt.tight_layout()
train_df = sns.load_dataset("titanic")
_ = sns.catplot(x='pclass', y='age', data=train_df, kind='box', height=8, ax=axes[0, 0])
_ = sns.catplot(x='embarked', y='age', data=train_df, kind='box', height=8, ax=axes[0, 1])
_ = sns.catplot(x='sex', y='age', data=train_df, kind='box', height=8, ax=axes[1, 0])
_ = sns.catplot(x='sex', y='age', hue='pclass', data=train_df, kind='box', height=8, ax=axes[1, 1])
_ = sns.catplot(x='sibsp', y='age', data=train_df, kind='box', height=8, ax=axes[2, 0])
_ = sns.catplot(x='parch', y='age', data=train_df, kind='box', height=8, ax=axes[2, 1])
# iterate over plots and run
# plt.close() to prevent duplicate
# subplot setup
var_dict = vars().copy()
var_keys = var_dict.keys()
plots_names = [x for x in var_keys if isinstance(var_dict[x], sns.axisgrid.FacetGrid)]
for p in plots_names:
plt.close(vars()[p].fig)
Plot 2:
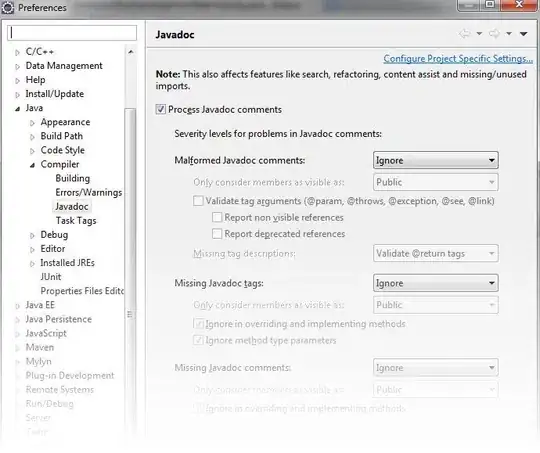