I have commented all the lines where I added my input. I hope this is what you were looking for.
Option 1 (Line plot):
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from matplotlib.ticker import MaxNLocator #imported this to set the tick locators correct
import seaborn as sns
%matplotlib inline
dic = {'Year': {0: 2004, 1: 2009, 2: 2014, 3: 2019}, 'BJP': {0: 40.67, 1: 35.2, 2: 46.63, 3: 56.56}, 'INC': {0: 54.81, 1: 57.11, 2: 15.22, 3: 22.63}, 'AAP': {0: 0.0, 1: 0.0, 2: 33.08, 3: 18.2}, 'Total_Polling': {0: 47.09, 1: 51.81, 2: 65.1, 3: 60.59}}
parl_data = pd.DataFrame(dic)
fig, ax = plt.subplots(figsize = (15,6)) # assigned fig and ax in one line and added size to figure for better visibility
plt.plot(parl_data['BJP'], marker='o', label='BJP', color='red')
plt.plot(parl_data['INC'], marker='o', label='INC', color='blue')
plt.plot(parl_data['AAP'], marker='o', label='AAP', color='brown')
plt.plot(parl_data['Total_Polling'], marker='o', label='Total Polling', color='green', linestyle='dashed')
plt.legend()
for i,j in parl_data.BJP.items():
ax.annotate(str(j), xy=(i, j), size = 15) # increased the font size as it was looking cluttered
for i,j in parl_data.INC.items():
ax.annotate(str(j), xy=(i, j), size = 15) # increased the font size as it was looking cluttered
for i,j in parl_data.AAP.items():
ax.annotate(str(j), xy=(i, j), size = 15) # increased the font size as it was looking cluttered
for i,j in parl_data.Total_Polling.items():
ax.annotate(str(j), xy=(i, j), size = 15) # increased the font size as it was looking cluttered
ax.set_alpha(0.8)
ax.set_title("\n\nParty-wise vote share in Lok Sabha Polls (Delhi) 2019\n", fontsize=15)
ax.set_ylabel("Parliament Polling Percentage\n", fontsize=15);
ax.set_xlabel("Election Year\n", fontsize=15)
plt.yticks(np.arange(0,100,10))
##I Added from here
ax.xaxis.set_major_locator(MaxNLocator(4)) # To set the locators correct
ax.set_xticklabels(['0','2004','2009','2014','2019']) # To set the labels correct.
plt.tick_params( left = False,bottom = False, labelsize= 13) #removed tick lines
plt.legend(frameon = False, loc = "best", ncol=4, fontsize = 14) # removed the frame around the legend and spread them along a line
plt.box(False) # Removed the box - 4 lines - you may keep if you like. Comment this line out
plt.style.use('bmh') #used the style bmh for better look n feel (my view) you may remove or keep as you like
plt.show();
It looks as below:
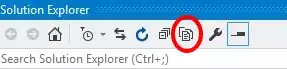
Option 2 (Bar plot + line):
width = .35
ind = np.arange(len(parl_data))
fig, ax = plt.subplots(figsize = (15,6)) # assigned fig and ax in one line and added size to figure for better visibility
#plot bars for bjp,inc & aap
bjp = plt.bar(ind,parl_data['BJP'],width/2, label='BJP', color='red')
inc = plt.bar(ind+width/2,parl_data['INC'],width/2, label='INC', color='green')
aap = plt.bar(ind+width,parl_data['AAP'],width/2, label='AAP', color='orange')
#Make a line plot for Total_Polling
plt.plot(parl_data['Total_Polling'],'bo-',label='Total Polling', color = 'darkred')
def anotate_bars(bars):
'''
This function helps annotate the bars with data labels in the desired position.
'''
for bar in bars:
h = bar.get_height()
ax.text(bar.get_x()+bar.get_width()/2., 0.75*h, h,ha='center', va='bottom', color = 'white', fontweight='bold')
anotate_bars(bjp)
anotate_bars(inc)
anotate_bars(aap)
for x,y in parl_data['Total_Polling'].items():
ax.text(x, y+4, y,ha='center', va='bottom', color = 'black', fontweight='bold')
ax.set_title("\n\nParty-wise vote share in Lok Sabha Polls (Delhi) 2019\n", fontsize=15)
ax.set_ylabel("Parliament Polling Percentage\n", fontsize=15);
ax.set_xlabel("Election Year\n", fontsize=15)
plt.yticks(np.arange(0,100,10))
ax.xaxis.set_major_locator(MaxNLocator(4)) # To set the locators correct
ax.set_xticklabels(['0','2004','2009','2014','2019']) # To set the labels correct.
plt.tick_params( left = False,bottom = False, labelsize= 13) #removed tick lines
plt.legend(frameon = False, loc = "best", ncol=4, fontsize = 14) # removed the frame around the legend and spread them along a line
plt.style.use('bmh') #used the style bmh for better look n feel (my view) you may remove or keep as you like
plt.show();
Output is as below:
