You can perform filtering in FB RTDB, it is not as sophisticated or flexible as Firestore (you can only filter on one key or value at once) but if it is as trivial as your example then it is certainly possible (you need to use indexing to improve performance and limit data download when in production).
On a db structure like this (ignore the nodes below 'root' that are not 'users', I use this 'root' node for multiple code tests during my development):
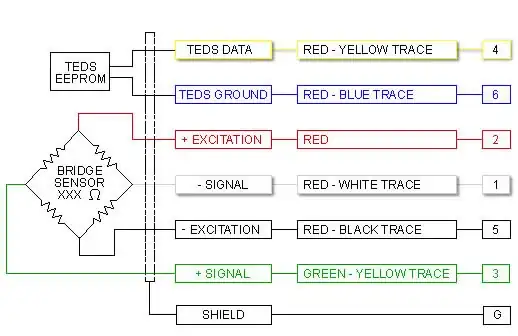
and using this code (in Flutter/Dart but the same parameters are available in other languages):
_referenceRoot
.child("root")
.child("users")
.orderByChild("gender")
.equalTo("male")
.once()
.then(
(DataSnapshot snapshot) {
print("snapshot.value: ${snapshot.value}");
if (snapshot.value != null) {
print("snapshot.key: ${snapshot.key}");
}
},
);
I get this output ie.only the male users. Note that in the snapshot the selected users are unordered:
I/flutter ( 4307): snapshot.value: {a2: {hair: brown, gender: male, age: 20}, a3: {hair: brown, gender: male, age: 20}, a4: {hair: brown, gender: male, age: 20}, a6: {hair: brown, gender: male, age: 20}, a8: {hair: brown, gender: male, age: 20}, a9: {hair: brown, gender: male, age: 20}, a0: {hair: brown, gender: male, age: 20}}
I/flutter ( 4307): snapshot.key: users
See this for more details of what is possible: https://firebase.google.com/docs/database/rest/retrieve-data
So, to answer your specific question, if the filtering you need to do is trivial, I would use only FB RTDB. If more complex, then will have to be Firestore. You would need to do the math on estimated data storage/download volumes vs. db calls and storage for a view on minimising costs.