You may be able to fix things the way you have them, but...
Instead of activating a second constraint (you're not showing where you're deactivating a showingCon
constraint), I'm going to suggest you use a single Height
constraint for your text view, and change the .constant
value...
Here's a very basic example that is working for me:
import UIKit
class ShrinkTextViewCell: UITableViewCell {
@IBOutlet var textViewHeightConstraint: NSLayoutConstraint!
@IBOutlet var theTextView: UITextView!
var tableUpdateCallback: (()->())?
@IBAction func didTap(_ sender: Any) {
if theTextView.alpha > 0.0 {
UIView.animate(withDuration: 0.5) {
self.theTextView.alpha = 0.0
self.textViewHeightConstraint.constant = 0
self.contentView.layoutIfNeeded()
self.tableUpdateCallback?()
}
} else {
UIView.animate(withDuration: 0.5) {
self.theTextView.alpha = 1.0
self.textViewHeightConstraint.constant = 128
self.contentView.layoutIfNeeded()
self.tableUpdateCallback?()
}
}
}
}
class ShrinkTextViewTableViewController: UITableViewController {
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return 5
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let c = tableView.dequeueReusableCell(withIdentifier: "ShrinkTextViewCell", for: indexPath) as! ShrinkTextViewCell
c.tableUpdateCallback = {
tableView.beginUpdates()
tableView.endUpdates()
}
return c
}
}
and here is the source of the Storyboard:
<?xml version="1.0" encoding="UTF-8"?>
<document type="com.apple.InterfaceBuilder3.CocoaTouch.Storyboard.XIB" version="3.0" toolsVersion="15505" targetRuntime="iOS.CocoaTouch" propertyAccessControl="none" useAutolayout="YES" useTraitCollections="YES" useSafeAreas="YES" colorMatched="YES" initialViewController="9iy-6q-gap">
<device id="retina4_7" orientation="portrait" appearance="light"/>
<dependencies>
<deployment identifier="iOS"/>
<plugIn identifier="com.apple.InterfaceBuilder.IBCocoaTouchPlugin" version="15510"/>
<capability name="documents saved in the Xcode 8 format" minToolsVersion="8.0"/>
</dependencies>
<scenes>
<!--Shrink Text View Table View Controller-->
<scene sceneID="oHl-Pu-bIJ">
<objects>
<tableViewController id="wSO-s3-dB0" customClass="ShrinkTextViewTableViewController" customModule="scratchy" customModuleProvider="target" sceneMemberID="viewController">
<tableView key="view" clipsSubviews="YES" contentMode="scaleToFill" alwaysBounceVertical="YES" dataMode="prototypes" style="plain" separatorStyle="default" rowHeight="-1" estimatedRowHeight="-1" sectionHeaderHeight="28" sectionFooterHeight="28" id="WtR-bt-uSy">
<rect key="frame" x="0.0" y="0.0" width="375" height="667"/>
<autoresizingMask key="autoresizingMask" widthSizable="YES" heightSizable="YES"/>
<color key="backgroundColor" systemColor="systemBackgroundColor" cocoaTouchSystemColor="whiteColor"/>
<prototypes>
<tableViewCell clipsSubviews="YES" contentMode="scaleToFill" preservesSuperviewLayoutMargins="YES" selectionStyle="default" indentationWidth="10" reuseIdentifier="ShrinkTextViewCell" rowHeight="189" id="gZx-b8-Hf3" customClass="ShrinkTextViewCell" customModule="scratchy" customModuleProvider="target">
<rect key="frame" x="0.0" y="28" width="375" height="189"/>
<autoresizingMask key="autoresizingMask"/>
<tableViewCellContentView key="contentView" opaque="NO" clipsSubviews="YES" multipleTouchEnabled="YES" contentMode="center" preservesSuperviewLayoutMargins="YES" insetsLayoutMarginsFromSafeArea="NO" tableViewCell="gZx-b8-Hf3" id="xmG-jI-EeI">
<rect key="frame" x="0.0" y="0.0" width="375" height="189"/>
<autoresizingMask key="autoresizingMask"/>
<subviews>
<textView clipsSubviews="YES" multipleTouchEnabled="YES" contentMode="scaleToFill" textAlignment="natural" translatesAutoresizingMaskIntoConstraints="NO" id="CGL-ug-wBr">
<rect key="frame" x="16" y="11" width="240" height="128"/>
<color key="backgroundColor" red="0.99953407049999998" green="0.98835557699999999" blue="0.47265523669999998" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<constraints>
<constraint firstAttribute="width" constant="240" id="Dip-pf-MUH"/>
<constraint firstAttribute="height" priority="999" constant="128" id="mK2-jh-d1y"/>
</constraints>
<string key="text">Lorem ipsum dolor sit er elit lamet, consectetaur cillium adipisicing pecu, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum. Nam liber te conscient to factor tum poen legum odioque civiuda.</string>
<color key="textColor" systemColor="labelColor" cocoaTouchSystemColor="darkTextColor"/>
<fontDescription key="fontDescription" type="system" pointSize="14"/>
<textInputTraits key="textInputTraits" autocapitalizationType="sentences"/>
</textView>
<button opaque="NO" contentMode="scaleToFill" contentHorizontalAlignment="center" contentVerticalAlignment="center" buttonType="roundedRect" lineBreakMode="middleTruncation" translatesAutoresizingMaskIntoConstraints="NO" id="vto-8g-NOW">
<rect key="frame" x="313" y="11" width="46" height="30"/>
<color key="backgroundColor" red="0.92143100499999997" green="0.92145264149999995" blue="0.92144101860000005" alpha="1" colorSpace="custom" customColorSpace="sRGB"/>
<state key="normal" title="Button"/>
<connections>
<action selector="didTap:" destination="gZx-b8-Hf3" eventType="touchUpInside" id="4nC-JL-rWD"/>
</connections>
</button>
</subviews>
<constraints>
<constraint firstAttribute="bottomMargin" relation="greaterThanOrEqual" secondItem="vto-8g-NOW" secondAttribute="bottom" id="5P3-E0-KJV"/>
<constraint firstItem="CGL-ug-wBr" firstAttribute="leading" secondItem="xmG-jI-EeI" secondAttribute="leadingMargin" id="6C9-8M-fUa"/>
<constraint firstItem="vto-8g-NOW" firstAttribute="top" secondItem="xmG-jI-EeI" secondAttribute="topMargin" id="Fwc-nY-zK8"/>
<constraint firstAttribute="trailingMargin" secondItem="vto-8g-NOW" secondAttribute="trailing" id="Gfq-Ps-hxG"/>
<constraint firstAttribute="bottomMargin" relation="greaterThanOrEqual" secondItem="CGL-ug-wBr" secondAttribute="bottom" id="PFA-sR-BPk"/>
<constraint firstItem="CGL-ug-wBr" firstAttribute="top" secondItem="xmG-jI-EeI" secondAttribute="topMargin" id="vyi-D5-OSn"/>
</constraints>
</tableViewCellContentView>
<connections>
<outlet property="textViewHeightConstraint" destination="mK2-jh-d1y" id="jgg-nH-KJ0"/>
<outlet property="theTextView" destination="CGL-ug-wBr" id="GRu-g4-TPK"/>
</connections>
</tableViewCell>
</prototypes>
<connections>
<outlet property="dataSource" destination="wSO-s3-dB0" id="hW0-YN-O65"/>
<outlet property="delegate" destination="wSO-s3-dB0" id="S0F-gf-dAv"/>
</connections>
</tableView>
<navigationItem key="navigationItem" id="HhZ-lH-fRh"/>
</tableViewController>
<placeholder placeholderIdentifier="IBFirstResponder" id="S7z-u4-GiE" userLabel="First Responder" customClass="UIResponder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="357.60000000000002" y="2048.7256371814096"/>
</scene>
<!--Navigation Controller-->
<scene sceneID="PyK-HU-aub">
<objects>
<navigationController automaticallyAdjustsScrollViewInsets="NO" id="9iy-6q-gap" sceneMemberID="viewController">
<toolbarItems/>
<navigationBar key="navigationBar" contentMode="scaleToFill" insetsLayoutMarginsFromSafeArea="NO" id="M2B-qr-tr5">
<rect key="frame" x="0.0" y="0.0" width="375" height="44"/>
<autoresizingMask key="autoresizingMask"/>
</navigationBar>
<nil name="viewControllers"/>
<connections>
<segue destination="wSO-s3-dB0" kind="relationship" relationship="rootViewController" id="ghB-H0-NIB"/>
</connections>
</navigationController>
<placeholder placeholderIdentifier="IBFirstResponder" id="AAD-E1-PrO" userLabel="First Responder" customClass="UIResponder" sceneMemberID="firstResponder"/>
</objects>
<point key="canvasLocation" x="-581.60000000000002" y="2048.7256371814096"/>
</scene>
</scenes>
</document>
The output looks like this:
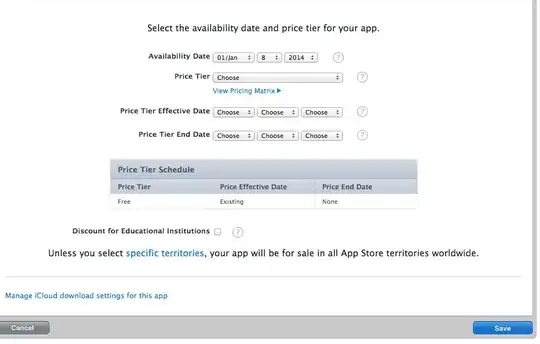
When you tap the button in each cell, the text view in that cell will "shrink and fade out" or "grow and fade in".