You can use the IndexOf
method to get the index of one string within another. It takes an argument that specifies where to start looking, so you can continually call it in a loop and just increment the starting index on each iteration. If it returns -1
, it means the string was not found, so we can use that as a condition for the loop.
For example, this method takes in a RichTextBox
control and a string
to search for, and it will highlight all instances of the search text within the RTB text:
private static void HighlightText(RichTextBox rtb, string text, Color? highlight = null)
{
if (rtb == null || rtb.TextLength == 0 || text == null || text.Length == 0) return;
// Find the first index of the text
var index = rtb.Text.IndexOf(text);
var length = text.Length;
var color = highlight ?? Color.Red; // Use Red if no color was specified
// While we found a match
while (index > -1)
{
// Highlight it
rtb.SelectionStart = index;
rtb.SelectionLength = length;
rtb.SelectionColor = color;
// Then try to find the next index of the text (starting after the previous one)
index = rtb.Text.IndexOf(text, index + length);
}
}
For sample usage, drop a RichTextBox
, a TextBox
, and a Button
control on the form, and add this code to the Button.Click
event:
private void button1_Click(object sender, EventArgs e)
{
HighlightText(richTextBox1, textBox1.Text);
}
Output
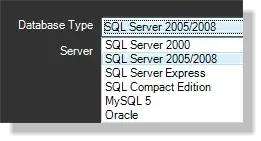
Update
If you want to highlight multiple items at once, you could create an overload of the method that takes in an array of strings and then calls the method above for each item in the array:
private static void HighlightItems(RichTextBox rtb, string[] items, Color? highlight = null)
{
if (items == null || items.Length == 0) return;
foreach (var item in items)
{
HighlightText(rtb, item, highlight);
}
}
A sample way to call this would be to take the text in textbox1
and split it on the semi-colon character. Then we can pass the resulting array to our overload method above:
private void button1_Click(object sender, EventArgs e)
{
// Un-highlight the text first
richTextBox1.SelectAll();
richTextBox1.SelectionColor = Color.Black;
// Call highlight with an array of strings created by
// splitting textbox1.Text on the ';' character
var multipleItems = textBox1.Text.Split(';');
HighlightItems(richTextBox1, multipleItems);
}
Output
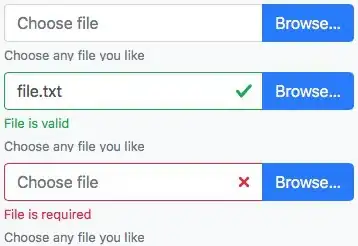