I've used a WKWebView to display a gif. The resulting view can be set anywhere. To set it as a background, you'll probably want to resize the contents of the WKWebView according to the contents of the superview.
import SwiftUI
import WebKit
struct HTMLRenderingWebView: UIViewRepresentable {
@Binding var htmlString: String
@Binding var baseURL: URL?
func makeUIView(context: Context) -> WKWebView {
let webView = WKWebView()
return webView
}
func updateUIView(_ uiView: WKWebView, context: Context) {
if self.htmlString != context.coordinator.lastLoadedHTML {
print("Updating HTML")
context.coordinator.lastLoadedHTML = self.htmlString
uiView.loadHTMLString(self.htmlString, baseURL: self.baseURL)
}
}
func makeCoordinator() -> Coordinator {
Coordinator(self)
}
class Coordinator: NSObject {
var parent: HTMLRenderingWebView
var lastLoadedHTML = ""
init(_ parent: HTMLRenderingWebView) {
self.parent = parent
}
}
}
struct HTMLRenderingWebViewExample: View {
@State var htmlString = ""
var body: some View {
VStack {
HTMLRenderingWebView(htmlString: self.$htmlString, baseURL: .constant(nil))
.padding(30).background(Color.gray)
Button("Click this button") {
self.htmlString = "<meta name=\"viewport\" content=\"initial-scale=1.0\" />" +
(self.assetAsString() ?? "image loading failed")
}
}.navigationBarTitle("Example HTML Rendering")
}
func assetAsString() -> String? {
let asset = NSDataAsset(name: "User_OhSqueezy_on_commons_wikimedia_org_Parallax_scrolling_example_scene")
if let data = asset?.data {
let base64string = data.base64EncodedString()
let format = "gif"
return "<img src='data:image/\(format);base64," + base64string + "' height=240 width=360>"
} else {
return nil
}
}
}
I got my animated gif from Wikimedia Commons and dragged it into the Assets.xcassets
in Xcode.
Result:
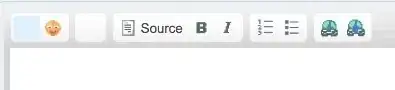