You may use the Console.SetWindowPosition()
method. *
Parameters
left
Int32
The column position of the upper left corner of the console window.
top
Int32
The row position of the upper left corner of the console window.
Here's an example to demonstrate:
static void Main(string[] args)
{
int i = 0;
while (true)
{
Console.ReadKey(false);
Console.WriteLine($"You are currently at line #{++i}");
Console.SetWindowPosition(0, i - 1);
}
}
Result:
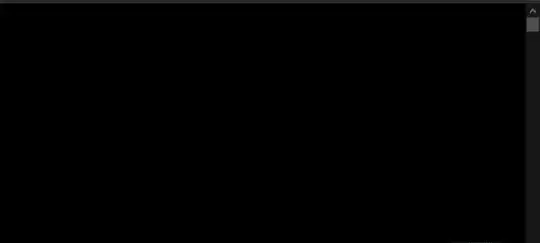
Note that if you're getting close to the value of Console.BufferHeight
, you could get an ArgumentOutOfRangeException, that is, when the new position (top) is greater than Console.BufferHeight - Console.WindowHeight
, so you might want to take that into account. I would add a simple condition to handle this case. Example:
int newTop = i - 1;
if (newTop + Console.WindowHeight <= Console.BufferHeight)
{
Console.SetWindowPosition(0, newTop);
}
..but you might want to handle this differently (e.g., increase the buffer as you go, change the window size, etc.)
* Don't be mislead by the name of the method. A "window" here doesn't mean the actual desktop/console window in OS terms (borders, etc.). Instead, it refers to the currently displayed window of the character buffer. To move the actual console window, you'd have to use the SetWindowPos
Windows API function. Example.