In OpenGL ES 1.1 you can't use glLineStipple
and you can't use a shader. But you can use a 1 dimensional texture and the Alpha test. See OpenGL ES 1.1 Full Specification.
OpenGL ES 1.1 does not support 1 dimensional textures, too. But that can be substituted by a 2 dimensional Nx1 texture, with ease.
Create 2D (4x1) texture with the internal format GL_ALPHA
and th following pattern:
1 0 0 1
The minifying and magnification function is GL_NEAREST
. The wrap parameters are GL_REPEAT
(that is default).
byte arr[] = new byte[] { 255, 0, 0, 255 };
ByteBuffer textureBuffer = ByteBuffer.wrap(arr);
gl2.glGenTextures(1, texture_id_, stippleTexObj);
gl.glBindTexture(GL.GL_TEXTURE_2D, stippleTexObj);
gl.glTexParameteri(GL.GL_TEXTURE_2D, GL.GL_TEXTURE_MIN_FILTER, GL.GL_NEAREST);
gl.glTexParameteri(GL.GL_TEXTURE_2D, GL.GL_TEXTURE_MAG_FILTER, GL.GL_NEAREST);
gl.glTexParameteri(GL.GL_TEXTURE_2D, GL.GL_TEXTURE_WRAP_S, GL.GL_REPEAT);
gl.glTexParameteri(GL.GL_TEXTURE_2D, GL.GL_TEXTURE_WRAP_T, GL.GL_REPEAT);
gl.glTexImage2D(GL.GL_TEXTURE_2D, 0, GL.GL_ALPHA, 4, 1, 0, GL.GL_ALPHA, GL.GL_UNSIGNED_BYTE, textureBuffer);
When you draw the lines, the you have to enable the Alpha test and enable 2 dimensional texturing.
gl.glEnable(GL11.GL_ALPHA_TEST);
gl.glAlphaFunc(GL11.GL_GEQUAL, 0.5f);
gl.glEnable(GL11.GL_TEXTURE_2D);
Ensure that the texture coordinates which are associated to the vertices are aligned to integral values when ypu draw the line :
e.g. a quad with bottom left of (-0.5 -0.5) and to right of (0.5, 0.5) and the texture coordinates in range [0, 5]:
x y u
-0.5f -0.5f 0.0f
0.5f -0.5f 5.0f
0.5f 0.5f 0.0f
-0.5f 0.5f 5.0f
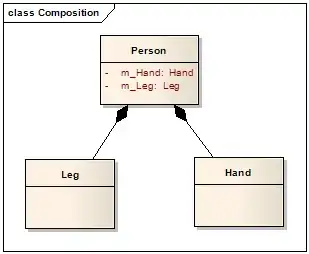