You can use the dataframe to read the edges directly into a NetworkX graph using from_pandas_adjacency. In order to do that, lets set the index of the dataframe
equal to chosen_currencies
, to ensure that the edges are mapped correctly.
from forex_python.converter import CurrencyRates
import pandas as pd
chosen_currencies = ['GBP', 'EUR', 'USD']
c = CurrencyRates()
rates = []
for currency_index in range(len(chosen_currencies)):
temp_list = []
for second_index in range(len(chosen_currencies)):
temp_list.append(c.get_rate(chosen_currencies[currency_index], chosen_currencies[second_index]))
rates.append(temp_list)
df = (pd.DataFrame.from_records(rates)).transpose()
df.columns = chosen_currencies
# GBP EUR USD
#0 1.000000 0.83238 0.768233
#1 1.201374 1.00000 0.922935
#2 1.301689 1.08350 1.000000
Now set the index
df.set_index([pd.Index(chosen_currencies)], inplace=True)
# GBP EUR USD
#GBP 1.000000 0.83238 0.768233
#EUR 1.201374 1.00000 0.922935
#USD 1.301689 1.08350 1.000000
Now let's create the graph
import networkx as nx
import matplotlib.pyplot as plt
G = nx.from_pandas_adjacency(df, create_using=nx.DiGraph)
# Set the figure size
plt.figure(figsize=(8,8))
# Get the edge labels and round them to 3 decimal places
# for more clarity while drawing
edge_labels = dict([((u,v), round(d['weight'], 3))
for u,v,d in G.edges(data=True)])
# Get the layout
pos = nx.spring_layout(G)
# Draw edge labels and graph
nx.draw_networkx_edge_labels(G,pos,edge_labels=edge_labels,
label_pos=0.15, font_size=10)
nx.draw(G, pos, with_labels=True,
connectionstyle='arc3, rad = 0.15',
node_size=800)
plt.show()
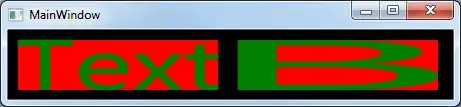
Note: The number near the arrowhead is the edge weight.
You can also view this Google Colab Notebook with above working example.
You can adjust the font size, label position, etc. according to your requirements.
References: