There's actually quite a few different ways to do this, but based on the code you've posted I'll assume you want to create your own geometry, using pixels as your coordinate system, and use that to paint a rectangle. This is one of those cases where WPF's flexibility means you have to be a lot more verbose than you would in previous platforms, but let's work through it...
The brick pattern can be achieved by creating a DrawingBrush containing a GeometryDrawing. The trick is to create two bricks of size <60,40> that are offset:
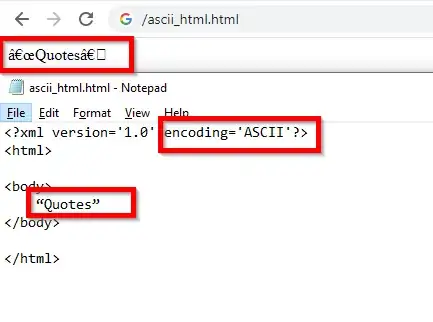
The problem now is the black background poking through. We can solve that by placing a third brick to the left of the one on the bottom, and then setting the DrawBrush's Viewport and Viewbox to the rectangle <0,0,60,80>, and then setting TileMode to "Tile". The code to do this is as follows:
var brickBrush = new DrawingBrush
{
Viewport = new Rect(0, 0, 60, 80),
ViewportUnits = BrushMappingMode.Absolute,
Viewbox = new Rect(0, 0, 60, 80),
ViewboxUnits = BrushMappingMode.Absolute,
TileMode = TileMode.Tile,
Drawing = new GeometryDrawing
{
Brush = new SolidColorBrush(Color.FromRgb(0xB1, 0x78, 0x33)),
Geometry = new GeometryGroup
{
Children = new GeometryCollection(new Geometry[] {
new RectangleGeometry(new Rect( 0, 0, 60, 40)),
new RectangleGeometry(new Rect( 30, 40, 60, 40)),
new RectangleGeometry(new Rect(-30, 40, 60, 40))
}),
},
Pen = new Pen(new SolidColorBrush(Color.FromRgb(0xE1, 0xD7, 0xBD)), 5)
}
};
theRectangle.Fill = brickBrush;
Which will generate the following pattern:
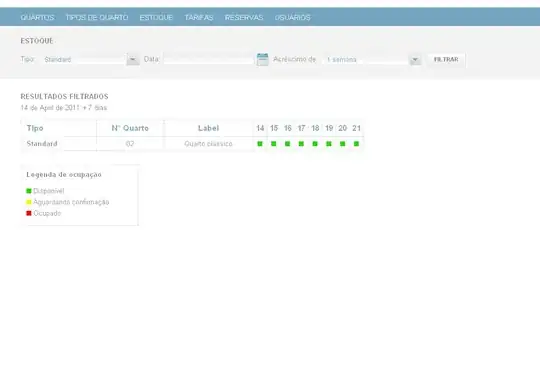
As your WPF skill improves you'll eventually want to start doing stuff like this in XAML. For the purpose of comparison, here is the code needed to achieve the same fill:
<Window.Resources>
<DrawingBrush x:Key="BrickBrush" Viewport="0,0,60,80" ViewportUnits="Absolute" Viewbox="0,0,60,80" ViewboxUnits="Absolute" TileMode="Tile">
<DrawingBrush.Drawing>
<GeometryDrawing Brush="#B17833">
<GeometryDrawing.Geometry>
<GeometryGroup>
<RectangleGeometry Rect="0,0,60,40" />
<RectangleGeometry Rect="30,40,60,40" />
<RectangleGeometry Rect="-30,40,60,40" />
</GeometryGroup>
</GeometryDrawing.Geometry>
<GeometryDrawing.Pen>
<Pen Thickness="5" Brush="#E1D7BD" />
</GeometryDrawing.Pen>
</GeometryDrawing>
</DrawingBrush.Drawing>
</DrawingBrush>
</Window.Resources>
<Rectangle Fill="{StaticResource BrickBrush}" />
You've also alluded to wanting diagonal bricks, to do that you simply add a rotation transform to the DrawingBrush:
var brickBrush = new DrawingBrush
{
Transform = new RotateTransform(45),
... etc ...
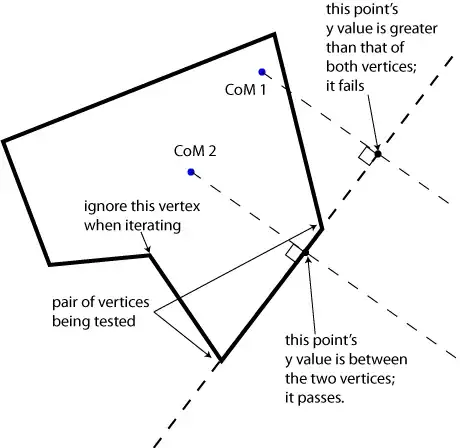