One approach...
- embed a
UIImageView
in a (green) UIView
- constrain the imageView on all 4 sides + 20-pts "padding"
- constrain the width of the greenView (or its leading and trailing)
- constrain the Y position of the greenView (top or centerY)
- constrain the height of the imageView with a multiplier based on the image width and height
Here is a simple example:
class ViewController: UIViewController {
let imgView: UIImageView = {
let v = UIImageView()
v.contentMode = .scaleToFill
return v
}()
let greenView: UIView = {
let v = UIView()
v.backgroundColor = .green
return v
}()
override func viewDidLoad() {
super.viewDidLoad()
// replace with your image name
guard let img = UIImage(named: "bkg640x360") else {
fatalError("Could not load image!")
}
view.backgroundColor = .black
// set the imgView's image
imgView.image = img
// use auto-layout constraints
imgView.translatesAutoresizingMaskIntoConstraints = false
greenView.translatesAutoresizingMaskIntoConstraints = false
// add imgView to greenview
greenView.addSubview(imgView)
// add greenView to self.view
view.addSubview(greenView)
// we want to respect safe-area
let g = view.safeAreaLayoutGuide
NSLayoutConstraint.activate([
// constrain greenView leading and trailing to view (safeArea)
greenView.leadingAnchor.constraint(equalTo: g.leadingAnchor, constant: 0.0),
greenView.trailingAnchor.constraint(equalTo: g.trailingAnchor, constant: 0.0),
// constrain greenView centerY to view centerY
greenView.centerYAnchor.constraint(equalTo: g.centerYAnchor, constant: 0.0),
// constrain imgView to all 4 sides of greenView with 20-pts "padding"
imgView.topAnchor.constraint(equalTo: greenView.topAnchor, constant: 20.0),
imgView.bottomAnchor.constraint(equalTo: greenView.bottomAnchor, constant: -20.0),
imgView.leadingAnchor.constraint(equalTo: greenView.leadingAnchor, constant: 20.0),
imgView.trailingAnchor.constraint(equalTo: greenView.trailingAnchor, constant: -20.0),
// constrain imgView proportional height equal to image height / width
imgView.heightAnchor.constraint(equalTo: imgView.widthAnchor, multiplier: img.size.height / img.size.width),
])
}
}
The result, using a 640 x 360
image:
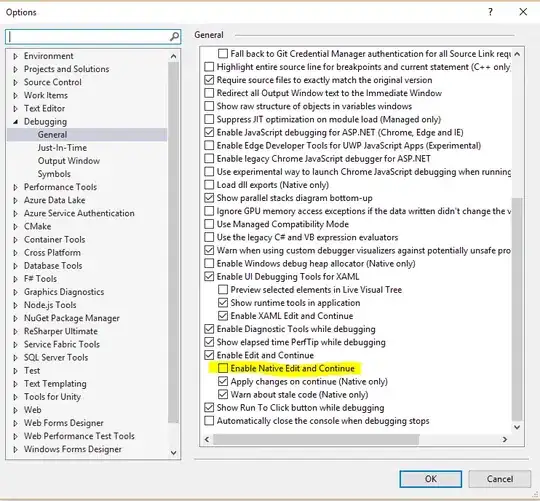
and using a 512 x 512
(square) image:
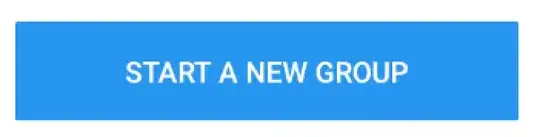
These are my source images:
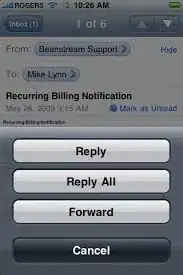
