New answer [will be in v1.47]
[It (Support \U \u \L \l replace modifiers) is in the Insiders' Build as of June 30, 2020 so it will be in 1.47 due out later in same week.]
(see https://github.com/microsoft/vscode/pull/96128: Initial implementation: Support \U \u \L \l replace modifiers).
Find/replace support for the case modifiers \U, \u, \L and \l is coming very soon. Their use is not supported yet in the search across files functionality but that is planned.
Find : (\w+\.\w+\.)(\w)(\w+)
Replace : $1get\u$2$3()
-- the PR to support \u
has been merged
See https://regex101.com/r/jZlO1D/1
For more on how the case modifiers work in vscode, see https://stackoverflow.com/a/62270300/836330
Just set up a snippet to do the replacement for you. Why? Because snippets can transform to upper and lower case and capitalize capture groups easily. Plus, although you don't need it here, they can also do conditional replacements which can be extremely powerful down the road for you.
Here is a simple snippet:
{
"key": "shift+alt+y", // whatever keybinding you choose
"command": "editor.action.insertSnippet",
"args": {
// see how simple this regex can be after you have found and selected your matches
"snippet": "${TM_SELECTED_TEXT/(.*\\.)(.*)/$1get${2:/capitalize}()/g}"
},
"when": "editorTextFocus && editorHasSelection"
},
You still have to find what you want to change, you can use your original regex if you want
\w+\.\w+\.(\w)(\w+)
I suggest (.+\.)([^\.\s]+)(?!.*\.)
see https://regex101.com/r/NbXgs3/2 it handles a few edge cases.
And allows you to have deeper structures like registerForm.main.subMain.myApplicant
, etc. up to any number or fewer like registerForm.myApplicant
.
- Step 1: Input your regex into the Find widget.
- Step 2:
Alt+Enter selects all your find
matches.
- Step 3: Trigger your snippet above, here
Shift+Alt+Y
Pretty easy. And then just keep the snippet around as a skeleton to use for future find/replaces with case or conditional transforms. Usually the interior regex - within the snippet, here (.*\\.)(.*)
- is relatively simple since it is only acting on your pre-selected entries already and thus the transform is simpler to grok.
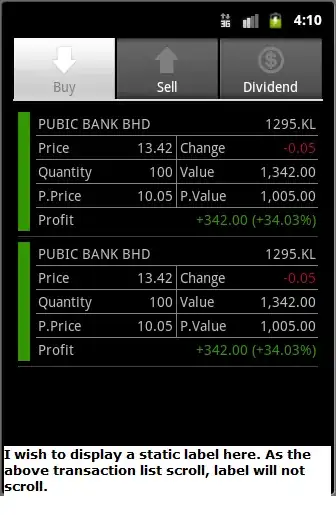