I haven't seen any answer describing how to get the current flavor directly from runtime config, after a lot of search I found this way:
For Android is quite simple, put this code in your MainActivity.kt:
private val CHANNEL = "flavor_channel"
override fun configureFlutterEngine(@NonNull flutterEngine: FlutterEngine) {
super.configureFlutterEngine(flutterEngine)
MethodChannel(flutterEngine.dartExecutor.binaryMessenger, CHANNEL).setMethodCallHandler {
call, result ->
when (call.method) {
"getFlavor" -> {
result.success(BuildConfig.FLAVOR)
}
}
}
}
For iOS, put that in the AppDelegate.swift:
let controller : FlutterViewController = window?.rootViewController as! FlutterViewController
let flavorChannel = FlutterMethodChannel(name: "flavor_channel",
binaryMessenger: controller.binaryMessenger)
flavorChannel.setMethodCallHandler({
(call: FlutterMethodCall, result: @escaping FlutterResult) -> Void in
// This method is invoked on the UI thread.
guard call.method == "getFlavor" else {
result(FlutterMethodNotImplemented)
return
}
self.receiveCurrentFlavor(result: result)
})
Then create this method at the bottom of AppDelegate class:
private func receiveCurrentFlavor(result: FlutterResult) {
var config: [String: Any]?
if let infoPlistPath = Bundle.main.url(forResource: "Info", withExtension: "plist") {
do {
let infoPlistData = try Data(contentsOf: infoPlistPath)
if let dict = try PropertyListSerialization.propertyList(from: infoPlistData, options: [], format: nil) as? [String: Any] {
config = dict
}
} catch {
print(error)
}
}
result(config?["Flavor"])
}
We now need to make the Flavor available in the infoDictionary. In Xcode, open Runner/Info.plist and add a key Flavor mapped to ${PRODUCT_FLAVOR}:
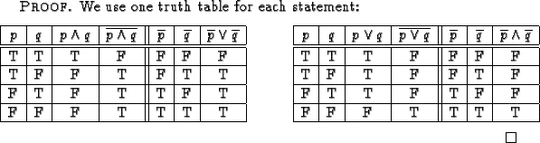
Now, under Targets/Runner/Build Settings/User-Defined and add a setting PRODUCT_FLAVOR. Add the flavor name for each configuration:

Then in Dart:
final flavor = await const MethodChannel("flavor_channel").invokeMethod("getFlavor");
Then you're done!