Use pandas.DataFrame.set_index
along with pandas.DataFrame.index
.
import pandas as pd
df = pd.DataFrame(
dict(
MUL1=["Data", "Data"],
MUL2=["Data", "Data"],
),
index=["Issue1", "Issue2"],
)
df = df.set_index("www." + df.index + ".com")
print(df)
edit: OP commented:
This just changes the index from 'issue1' to 'www.issue1.com' but does not make it clickable. (=HYPERLINK) – Aditya Garg
My response is that the clickabilty of the link depends on what program you are displaying the link in. I use Pycharm, which is able to detect URLs in console output and makes them clickable by default:
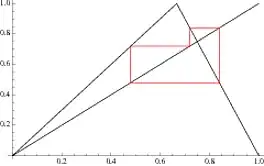
That being said it may not be clickable when running the script in other programs like cmd.exe for example. Making it into an html or markdown style link is not going to change this for things like cmd. However, if you're going to display this DataFrame in something that does support a particular link formatting method, then you can try some of the following examples:
import pandas as pd
df = pd.DataFrame(
dict(
MUL1=["Data", "Data"],
MUL2=["Data", "Data"],
),
index=["Issue1", "Issue2"],
)
index = df.index
# Basic link example
df = df.set_index('www.' + index + '.com/')
print(df)
# Markdown Link Example
df = df.set_index("[" + index + "](www." + index + ".com)")
print(df)
# angle bracket format example
df = df.set_index("<www." + index + ".com>")
print(df)
# html link example
df = df.set_index('<a href="www.' + index + '.com">' + index + '</a>')
print(df)