FWIW here's a simple example of plotting some values against times using the excellent and well-documented matplotlib:
data.csv:
VISIT_TIME TOTAL_VISITS
06:00:00 290
06:30:00 306
07:00:00 364
07:30:00 363
08:00:00 469
08:30:00 436
09:00:00 449
09:30:00 451
10:00:00 524
10:30:00 506
11:00:00 613
11:30:00 585
12:00:00 620
12:30:00 529
13:00:00 588
13:30:00 545
Simple program for illustrative purposes:
import matplotlib.dates as mdates
import matplotlib.mlab as mlab
import matplotlib.pyplot as plt
import datetime as dt
import sys
def main( datafile ):
np_rec_array = mlab.csv2rec( datafile, delimiter='\t' )
np_rec_array.sort() # in-place sort
# a `figure` is a starting point for MPL visualizations
fig = plt.figure( figsize=(8,6) )
# add a set of `axes` to above `figure`
ax = fig.add_subplot(111)
x = np_rec_array.visit_time
y = np_rec_array.total_visits
# `plot_date` is like `plot` but allows for easier x-axis formatting
ax.plot_date(x, y, 'o-', color='g')
# show time every 30 minutes
ax.xaxis.set_major_locator( mdates.MinuteLocator(interval=30) )
# specify time format
ax.xaxis.set_major_formatter( mdates.DateFormatter("%H:%M") )
# set x-axis label rotation (otherwise they can overlap)
for l in ax.get_xticklabels():
l.set_rotation(60)
plt.title( 'Website Visits' )
plt.show()
if __name__ == '__main__':
if len( sys.argv ) == 1:
sys.stderr.write( 'need a filename, exiting...' )
sys.exit(-1)
main( sys.argv[1] )
Output is the following image:
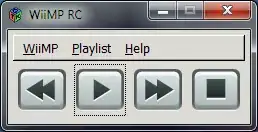