First import pandas and read csv through it and store the data into a dataframe object.
Use the .str.split
method to split the "Sentiment" column into two by the comma.
Then make new columns, remove the front and back brackets of the string value if any using .str.strip
.
Print the data. Or if you would like, write it to a new csv file using the .to_csv
method.
Remember to rename the csv file names in the .read_csv
and .to_csv
methods.
Complete Code:
import pandas as pd
# reading csv
data = pd.read_csv("file.csv")
# new data frame with split value columns
splitData = data["Sentiment"].str.split(",", n=1, expand=True)
# making new column Pos from first part of the split data,
# also remove front and back brackets if any
data["Pos"] = splitData[0].str.strip("()")
# making new column state from second part of the split data,
# also remove front and back brackets if any
data["state"] = splitData[1].str.strip("()")
# print data
print(data)
# write back to a new csv file
data.to_csv('newFile.csv')
Below are the outputs using mock data:
Print to Terminal:
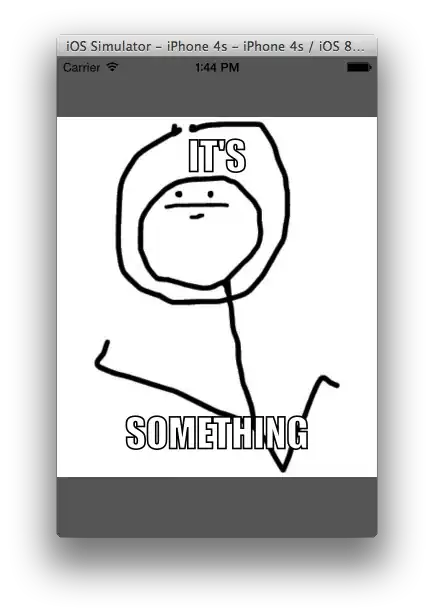
New CSV:
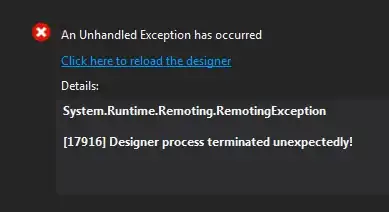