Make the view controller be the delegate of the text field, and implement the following methods:
extension ViewController: UITextFieldDelegate {
func textFieldDidChangeSelection(_ textField: UITextField) {
if textField.text?.isEmpty == true {
textField.text = "0"
}
}
func textField(_ textField: UITextField, shouldChangeCharactersIn range: NSRange, replacementString string: String) -> Bool {
if textField.text?.isEmpty == true && string.isEmpty {
textField.text = "0"
return false
} else if textField.text == "0" {
textField.text = string
return false
}
return true
}
}
If the user deletes a text entirely, the text field will show "0".
If the user starts typing with the initial "0" value, the "0" will disappear and show the user-typed text.
Edit: To set a view controller a delegate of the text field, right click (or ctrl + click) and drag from the textfield to the view controller in the storyboard:
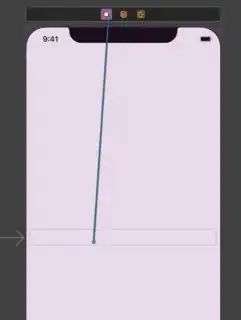
and choose delegate:
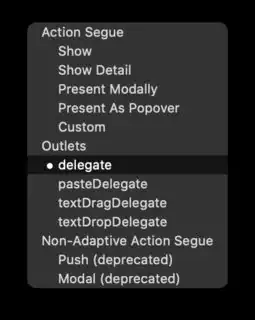
If you are not using a storyboard or don't want to use it, you can always do it programmatically.
override func viewDidLoad() {
super.viewDidLoad()
textField.delegate = self
}