IntStream
Your Question is unclear about data types and whether the value of the map entries should be null. I will presume the simple case of Integer
keys and null values.
Stream populates a map
The Random
and ThreadLocalRandom
classes now offer an ints
method. That method returns a stream of numbers, specifically a IntStream
.
int initialCapacity = 3; // 16_200 or 64_800 or 259_200.
Map < Integer, UUID > map = new HashMap <>( initialCapacity );
IntStream numbers = ThreadLocalRandom.current().ints( initialCapacity );
numbers.forEach( number -> map.put( number , null ) ); // Auto-boxing wraps the primitive `int` as an `Integer` object.
Dump to console.
System.out.println( map );
When run.
{837992347=null, 1744654283=null, -393617722=null}
Stream instantiates a map
You could even make a one-liner of this, asking the stream to produce the Map
instance rather than pre-initializing it.
I am not suggesting this is better, but it is a bit interesting. I had to ask Produce a Map from an IntStream of keys to learn how to do this.
Map < Integer, UUID > map =
ThreadLocalRandom
.current() // Returns a random number generator.
.ints( 3 ) // Produces three random numbers, the limit being the number passed here.
.boxed() // Invokes auto-boxing to wrap each primitive `int` as an `Integer` object.
.collect( // Gathers the outputs of this stream into a collection.
Collectors.toMap( // Returns a `Collector`, a collector that knows how to produce a `java.util.Map` object.
Function.identity() , // Returns each `Integer` produced by the stream. No further actions needed here, we just want the number.
number -> UUID.randomUUID() // Generate some object to use as the value in each map entry being generated. Not important to this demo.
) // Returns a `Collector` used by the `collect` method.
); // Returns a populated map.
map.toString(): {1996421820=bbb468b7-f789-44a9-bd74-5c3d060081d0, -717171145=7bd89a89-8b04-4532-82ae-437788378814, 996407145=351f3cdd-6e37-4a09-8644-59d846a815d4}
This stream-instantiates-map approach is the wrong way to go if you want to choose the concrete class implementing Map
.
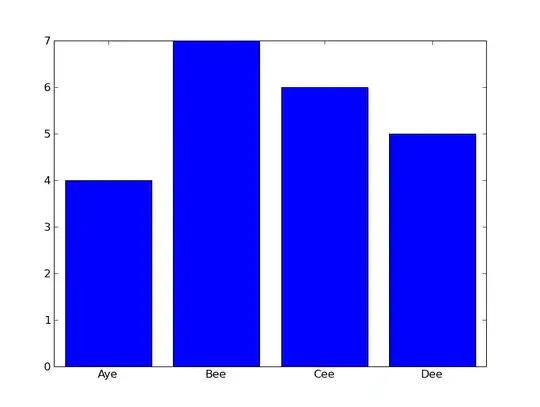