After your commented clarification and the addition of the Workbook
to your question, I've edited this Answer to reflect these updates.
Assuming you know the cell address for each value on sheet1
AND the cell address are constant.
I've written a Subroutine
to capture the values of your BBU Quote Entry
form and write them to your BBU Quote Database
range. I've added this to Module4
.
It should be noted, the code only works with the Basic Information section of your form AND the 2 option buttons for Hazardous
or Non-Hazardous
using a function ReturnFormControlCaption
. You can put in the hard yards for the rest of the data (more or less just copy paste, rename variables, adjust the range values and add the variables to the array of course).
Sub BBUEntryToDatabase()
Dim CustCompany As String
Dim CustName As String
Dim CustLocation As String
Dim CMTRep As String
Dim QuoteNo As String
Dim QuoteDate As String
Dim Hazard as String
With ThisWorkbook.Sheets("BBU Quote Entry")
CustCompany = .Range("D6").Value
CustName = .Range("D8").Value
CustLocaction = .Range("D10").Value
CMTRep = .Range("G6").Value
QuoteNo = .Range("G8").Value
QuoteDate = .Range("G10").Value
Hazard = ReturnFormControlCaption("BBU Quote Entry", "HazardousButton", "NotHazardousButton")
End With
Dim BBUArray As Variant
'The Array is assigned in order of your headings on "BBU Quote Database" sheet
BBUArray = Array(QuoteNo, CustCompany, CustName, CustLocation, CMTRep, QuoteDate, _
"Clearance", "Height", "Material", "Density", Hazard)
Dim Destination As Range
Dim LastRow As Long
With ThisWorkbook.Sheets("BBU Quote Database")
LastRow = .Cells(.Rows.Count, 2).End(xlUp).Row + 1
Set Destination = .Cells(LastRow, 2)
Set Destination = Destination.Resize(1, UBound(BBUArray, 1) + 1) ' + 1 as the array is 0 based (whereas columns start at 1).
Destination.Value = BBUArray
End With
End Sub
Here is a screenshot of my data entry
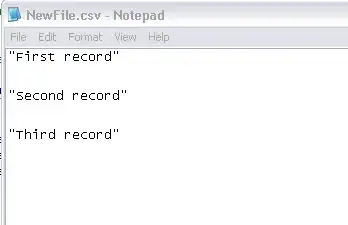
And the output on "BBU Quote Database" (after 3 tests with the same inputs)
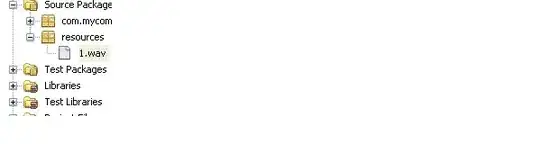
I'm not very familiar with Form Controls
as I usually use ActiveX Controls
which I find a bit easier to use with VBA - I'd assume there is probably a much cleaner way to deal with the OptionButtons
.
The ReturnFormControlCaption()
function:
Function ReturnFormControlCaption(ByVal SheetNameTheControlIsOn As String, ByVal FirstFormControlName As String, _
Optional ByVal SecondFormControlName As String, Optional ByVal ThirdFormControlName As String, _
Optional ByVal FourthFormControlName As String, Optional ByVal FifthFormControlName As String, _
Optional ByVal SixthFormControlName As String) As String
With ThisWorkbook.Sheets(SheetNameTheControlIsOn)
If SecondFormControlName = "" Then
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not Specified"
End If
ElseIf ThirdFormControlName = "" Then
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SecondFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SecondFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not specified"
End If
ElseIf FourthFormControlName = "" Then
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SecondFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SecondFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(ThirdFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(ThirdFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not specified"
End If
ElseIf FifthFormControlName = "" Then
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SecondFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SecondFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(ThirdFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(ThirdFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(FourthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FourthFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not specified"
End If
ElseIf SixthFormControlName = "" Then
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SecondFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SecondFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(ThirdFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(ThirdFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(FourthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FifthFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(FifthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FifthFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not specified"
End If
Else
If .Shapes(FirstFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FirstFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SecondFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SecondFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(ThirdFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(ThirdFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(FourthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FifthFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(FifthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(FifthFormControlName).OLEFormat.Object.Caption
ElseIf .Shapes(SixthFormControlName).OLEFormat.Object.Value = 1 Then
ReturnFormControlCaption = .Shapes(SixthFormControlName).OLEFormat.Object.Caption
Else
ReturnFormControlCaption = "Not specified"
End If
End If
End With
End Function
To briefly explain the function, you pass string
variables for the relevant worksheet name, and at least one (up to six) form control name(s).
The lengthy and nested If...ElseIf...Else
statements are first establishing up to which argument has been included. Then depending on which argument is the first empty or ""
value, it executes the next If...ElseIf...Else
statement to determine in this case, which OptionButton
is selected and then returns the .Caption
of that OptionButton
.
If none of the OptionButton
being evaluated are selected, it returns "Not specified".
Note: This function will work for determining which CheckBox
is checked BUT if in your passed arguments, more than one is selected, it will only return the .Caption
of the first CheckBox
that is checked. With some modification you could get the function working for both types including all CheckBox
being checked.
Chip Pearson has some excellent information about Arrays and how to use them. You can read up on them on his website at www.cpearson.com or specifially what we have done here with arrays on this article on his website