You will find hardware disk manufacture name, for example, at the following Registry key:
HKEY_LOCAL_MACHINE\HARDWARE\DEVICEMAP\Scsi\Scsi Port 0\Scsi Bus 0\Target Id 0\Logical Unit Id 0
There is a value name "Identifier
" seems what you are looking for:
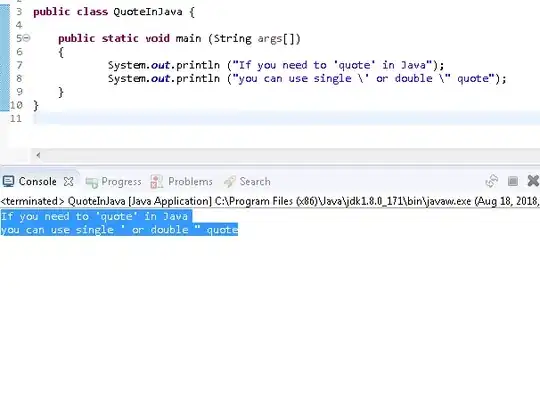
The following is an example for query this value using Registry Functions:
#include <windows.h>
#include <tchar.h>
#define MAX_VALUE_NAME 16383
void QueryKey(HKEY hKey)
{
DWORD cValues; // number of values for key
DWORD retCode;
TCHAR pvData[MAX_VALUE_NAME];
DWORD cbData = sizeof(TCHAR) * MAX_VALUE_NAME;
TCHAR targetValue[] = L"Identifier";
// Get the value count.
retCode = RegQueryInfoKey(
hKey, // key handle
NULL,
NULL,
NULL,
NULL,
NULL,
NULL,
&cValues, // number of values for this key
NULL,
NULL,
NULL,
NULL);
// Get the key value.
if (cValues)
{
retCode = RegGetValue(hKey, NULL, targetValue, RRF_RT_REG_SZ, NULL, pvData, &cbData);
if (retCode != ERROR_SUCCESS)
{
_tprintf(TEXT("RegGetValue fails with error: %d\n", retCode));
return;
}
_tprintf(TEXT("%s: %s\n"), targetValue, pvData);
}
}
void main(void)
{
HKEY hTestKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("HARDWARE\\DEVICEMAP\\\Scsi\\Scsi Port 0\\Scsi Bus 0\\Target Id 0\\Logical Unit Id 0"),
0,
KEY_READ,
&hTestKey) == ERROR_SUCCESS
)
{
QueryKey(hTestKey);
}
RegCloseKey(hTestKey);
}