You have to use Apache Commons CSV
to read .csv
files.
Download the jar from here - https://commons.apache.org/proper/commons-csv/download_csv.cgi
You can try this :
I created an .csv file in my local - Book1.csv
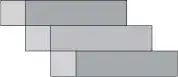
You should modify the column to make your work easier.
Select the all the rows of the column and right click and click on Format cells.

Click on number tab -> Click on Custom menu and select -> hh:mm:ss
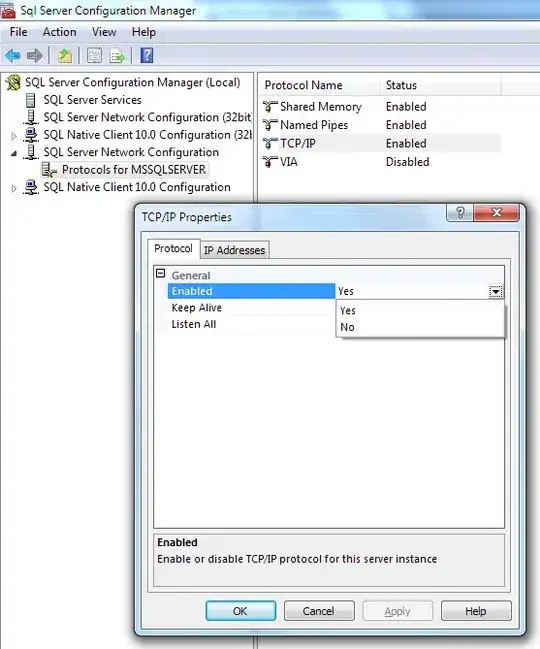
After this Click on OK to get the changes.
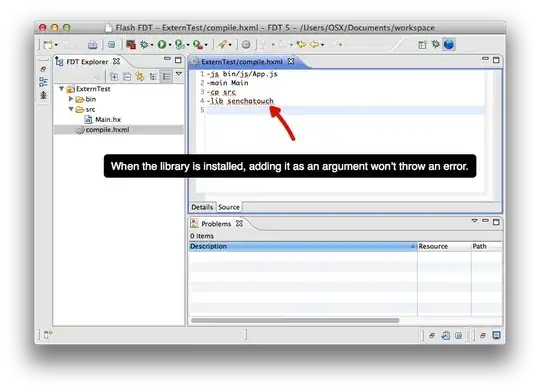
The code :
public class Testing {
public static void main(String args[]) throws ParseException {
try (Reader reader = new FileReader("C:\\Users\\Anish\\Desktop\\Book1.csv");
CSVParser csvParser = new CSVParser(reader, CSVFormat.DEFAULT);) {
System.out.println("Loaded");
for (CSVRecord csvRecord : csvParser.getRecords()) {
SimpleDateFormat format = new SimpleDateFormat("hh:mm:ss");
Date date = format.parse(csvRecord.get(0));
System.out.println(date.getHours() + ":" + date.getMinutes() + ":" + date.getSeconds());
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Output :
Loaded
0:44:11
0:29:4
0:22:41