This error occurs when you perform calculations with variables that use letters combined with numbers (alphanumeric), for example 24kb, 886ab ...
I had the error in the following function
function get_config_bytes($val) {
$val = trim($val);
$last = strtolower($val[strlen($val)-1]);
switch($last) {
case 'g':
$val *= 1024;
case 'm':
$val *= 1024;
case 'k':
$val *= 1024;
}
return $this->fix_integer_overflow($val);
}
The application uploads images but it didn't work, it showed the following warning:
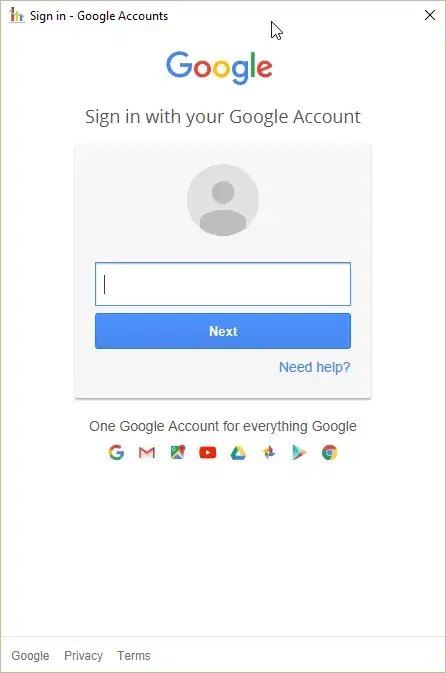
Solution: The intval()
function extracts the integer value of a variable with alphanumeric data and creates a new variable with the same value but converted to an integer with the intval()
function. Here is the code:
function get_config_bytes($val) {
$val = trim($val);
$last = strtolower($val[strlen($val)-1]);
$intval = intval(trim($val));
switch($last) {
case 'g':
$intval *= 1024;
case 'm':
$intval *= 1024;
case 'k':
$intval *= 1024;
}
return $this->fix_integer_overflow($intval);
}
The function fix_integer_overflow
// Fix for overflowing signed 32 bit integers,
// works for sizes up to 2^32-1 bytes (4 GiB - 1):
protected function fix_integer_overflow($size) {
if ($size < 0) {
$size += 2.0 * (PHP_INT_MAX + 1);
}
return $size;
}