I would like to mention other two very interesting and powerful libraries:
- pymeshlab: a Python library that interfaces to MeshLab, the popular open source application for editing and processing large 3D triangle meshes.
- pyvista: a high-level Python API to the Visualization Toolkit (VTK).
Both libraries allows to perform boolean operations between meshes. Let's consider the intersection between the Stanford bunny (~86k triangles) and a sphere:
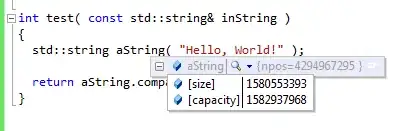
The code below compare the intersection and computational time of the two libraries.
from time import perf_counter
import pymeshlab
import pyvista as pv
import trimesh
bunny_path = "bunny.ply"
sphere_path = "sphere.ply"
def pymeshlab_intersection() -> None:
ms = pymeshlab.MeshSet()
ms.load_new_mesh(bunny_path)
ms.load_new_mesh(sphere_path)
ms.generate_boolean_intersection()
ms.save_current_mesh("pymeshlab_intersection.ply")
intersection_volume = ms.get_geometric_measures()["mesh_volume"]
print(f"pymeshlab - intersection volume = {intersection_volume}")
def pyvista_intersection() -> None:
bunny = trimesh.load_mesh(bunny_path, process=False, maintain_order=True)
bunny_pv = pv.wrap(bunny)
sphere = trimesh.load_mesh(sphere_path, process=False, maintain_order=True)
sphere_pv = pv.wrap(sphere)
intersection = bunny_pv.boolean_intersection(sphere_pv)
intersection.save("pyvista_intersection.ply")
print(f"pyvista - intersection volume = {intersection.volume}")
if __name__ == "__main__":
t0 = perf_counter()
pymeshlab_intersection()
print(f"pymeshlab: {perf_counter() - t0} s")
t0 = perf_counter()
pyvista_intersection()
print(f"pyvista: {perf_counter() - t0} s")
pymeshlab:
- intersection volume:
14799.202211491009
- elapsed time:
2.1220125 s
pyvista:
- intersection volume:
14799.297891938557
- elapsed time:
4.3342883 s
So, pymeshlab is twice as fast as pyvista.
Below the resulting mesh created by pymeshlab:
